Introduction to Tensors
Tensors are fundamental data structures in the field of machine learning and deep learning, serving as the building blocks for building and training models. A tensor is a multi-dimensional array that extends the concept of scalars, vectors, and matrices, allowing us to represent complex data in a structured format. In essence, while a scalar is a zero-dimensional tensor, a vector is a one-dimensional tensor, and a matrix is a two-dimensional tensor, higher-dimensional tensors are essentially the logical extension that allows for more sophisticated data representation.
The significance of understanding tensors in PyTorch is underscored by their versatile application across various computational tasks. Tensors can represent anything from a single data point to batches of images or even multi-dimensional datasets. This flexibility enables deep learning frameworks, like PyTorch, to perform extensive mathematical operations seamlessly, facilitating the training of neural networks with large datasets.
It is crucial to distinguish tensors from traditional arrays and matrices, which are limited mainly in terms of dimensions and operations. While arrays and matrices predominantly focus on numerical data, tensors can accommodate various data types, making them ideal for complex computations necessary in machine learning tasks. PyTorch’s tensor library offers improved performance through GPU acceleration, allowing for faster calculations, which is indispensable in the context of big data processing and model training.
As we delve deeper into this guide, we will explore the functionalities of tensors specifically within the PyTorch framework. By understanding tensors in PyTorch with hands-on examples, practitioners will gain practical skills necessary for efficient model designing and implementation. This foundational knowledge will be pivotal as we progress to more advanced topics related to tensor operations and manipulations.
Why Use PyTorch for Tensors?
PyTorch has emerged as one of the leading frameworks for tensor operations, and this popularity can be attributed to several key advantages it offers to researchers and developers alike. First and foremost, PyTorch is known for its remarkable flexibility. Unlike many other frameworks that require static computation graphs, PyTorch employs a dynamic computation graph model. This characteristic allows users to modify network behavior on-the-fly without the overhead of recompilation. Consequently, this dynamic approach aligns seamlessly with the iterative nature of many machine learning experiments, promoting a more intuitive and faster development process.
Another significant advantage of using PyTorch for tensor operations is its ease of use. PyTorch’s syntax is designed to be clear and Pythonic, making it accessible for both newcomers and seasoned practitioners. This user-friendly structure encourages rapid prototyping: developers can implement their tensor operations efficiently and with minimal boilerplate code. Furthermore, this ease of use extends to debugging, as the dynamic graphs enable real-time error tracking and adjustments.
Moreover, PyTorch benefits from strong community support and extensive documentation, which aids in the learning curve. Developers can find a wealth of tutorials, forums, and resources dedicated to understanding tensors in PyTorch with hands-on examples, thus enhancing their proficiency in applying the framework to various challenges. In addition, the seamless integration with popular libraries like NumPy and SciPy further extends its capabilities and usability.
In summary, the advantages of PyTorch in the realm of tensor operations, including its flexibility through dynamic computation graphs and user-friendly design, make it a preferred choice for many practitioners. Its community support and extensive capabilities facilitate a deeper understanding of tensors in PyTorch with hands-on examples, ultimately leading to more effective and efficient outcomes in machine learning projects.
Creating Tensors in PyTorch
Creating tensors in PyTorch is an essential step that serves as the foundation for performing various computations, including deep learning tasks. PyTorch provides several functions to facilitate this process, each catering to specific requirements. Understanding tensors in PyTorch with hands-on examples is crucial for deploying them effectively.
The simplest way to create a tensor is by using the torch.tensor()
function. This function takes an existing data structure, such as a list or a NumPy array, and converts it into a tensor. For instance, executing torch.tensor([[1, 2], [3, 4]])
will yield a 2×2 tensor containing the provided values. It is important to note that this method infers the data type of the tensor from the input data.
Another common method is to use torch.zeros()
and torch.ones()
, both of which generate tensors filled with zeros and ones, respectively. For example, torch.zeros((3, 4))
creates a 3×4 tensor filled with zeros, making it useful for initializing weights in neural networks. Conversely, torch.ones((2, 3))
results in a 2×3 tensor filled with ones, which is particularly handy in certain mathematical computations.
Additionally, one can leverage the torch.rand()
function to generate tensors with values uniformly distributed between 0 and 1. Using torch.rand(2, 2)
creates a 2×2 tensor populated with random float values. Each of these methods provides a straightforward approach to creating tensors tailored to specific applications, reinforcing the importance of understanding tensors in PyTorch with hands-on examples for practical implementation.
Tensor Operations: Basic Mathematical Functions
Tensors serve as the foundational data structure in PyTorch, facilitating mathematical computations essential for machine learning tasks. Understanding tensors in PyTorch with hands-on examples begins with basic tensor operations, which include addition, subtraction, multiplication, and division. These operations can be performed seamlessly with the built-in PyTorch functions, allowing users to manipulate data efficiently.
To begin, addition of tensors can be accomplished using the torch.add
function. For instance, if we have two tensors, A
and B
, defined as A = torch.tensor([1, 2, 3])
and B = torch.tensor([4, 5, 6])
, we can compute the sum by calling torch.add(A, B)
. The output will yield a new tensor containing the results of element-wise addition, demonstrating the elementary yet powerful arithmetic capabilities of tensors in PyTorch.
Similarly, subtraction is executed using the torch.sub
function. Given the same tensors A
and B
, performing the operation torch.sub(A, B)
results in a tensor that contains the differences between corresponding elements. The representation of mathematical operations over tensors illustrates the straightforward syntax utilized in PyTorch.
When it comes to multiplication, the torch.mul
function allows users to perform element-wise multiplication, and especially when dealing with two-dimensional tensors, the transitive property comes into play. PyTorch also supports matrix operations, where matrix product is computed through torch.mm
. Division, handled by the torch.div
function, also follows a similar format, providing users with a robust toolset for managing tensor-based calculations.
Hands-on examples involving these basic tensor operations not only exemplify the functionality of PyTorch but also enhance the user’s understanding of tensors in PyTorch with hands-on examples. Mastering these fundamental operations lays the groundwork for more advanced tensor manipulations and machine learning practices.
Indexing, Slicing, and Reshaping Tensors
Indexing and slicing tensors in PyTorch is a fundamental skill essential for effective tensor manipulation. Tensors are multi-dimensional arrays, and the ability to extract specific elements or sub-arrays is key to data processing and analysis. In PyTorch, the indexing syntax is similar to that of NumPy, which makes it easy for users familiar with that library to transition to PyTorch.
For instance, to index a tensor, you can simply use square brackets. Given a 2D tensor (matrix), you can index it as follows: tensor[row, column]
. If we have a tensor defined as data = torch.tensor([[1, 2], [3, 4]])
, accessing data[0, 1]
would yield the value 2
. Slicing allows for selecting ranges of data by using the colon (:) operator. For example, data[0, :]
extracts the entire first row of the tensor.
Advanced indexing techniques enable more complex data selections. Using boolean tensors or lists of indices can facilitate selecting multiple non-contiguous elements. For instance, if you have an index list [0, 1]
, applying it to a tensor will retrieve the corresponding elements based on the specified indices.
Reshaping tensors is another crucial aspect of tensor manipulation in PyTorch. Functions such as view()
and reshape()
allow for altering the shape of a tensor while maintaining its data. The view()
function requires the new shape’s dimensions to multiply back to the original size, enabling efficient memory usage. For example, transforming a tensor of size 6
to shape (2, 3)
can be performed with data.view(2, 3)
. Conversely, reshape()
offers greater flexibility as it can infer dimensions automatically.
By mastering indexing, slicing, and reshaping tensors, one can vastly enhance their proficiency in understanding tensors in PyTorch with hands-on examples, allowing for more effective data manipulation processes.
Tensor Broadcasting
Tensor broadcasting is a powerful feature in PyTorch that allows operations on tensors of different shapes, effectively expanding their dimensions to make them compatible for various mathematical operations. This capability improves computational efficiency and simplifies coding by eliminating the need for explicit tensor replication.
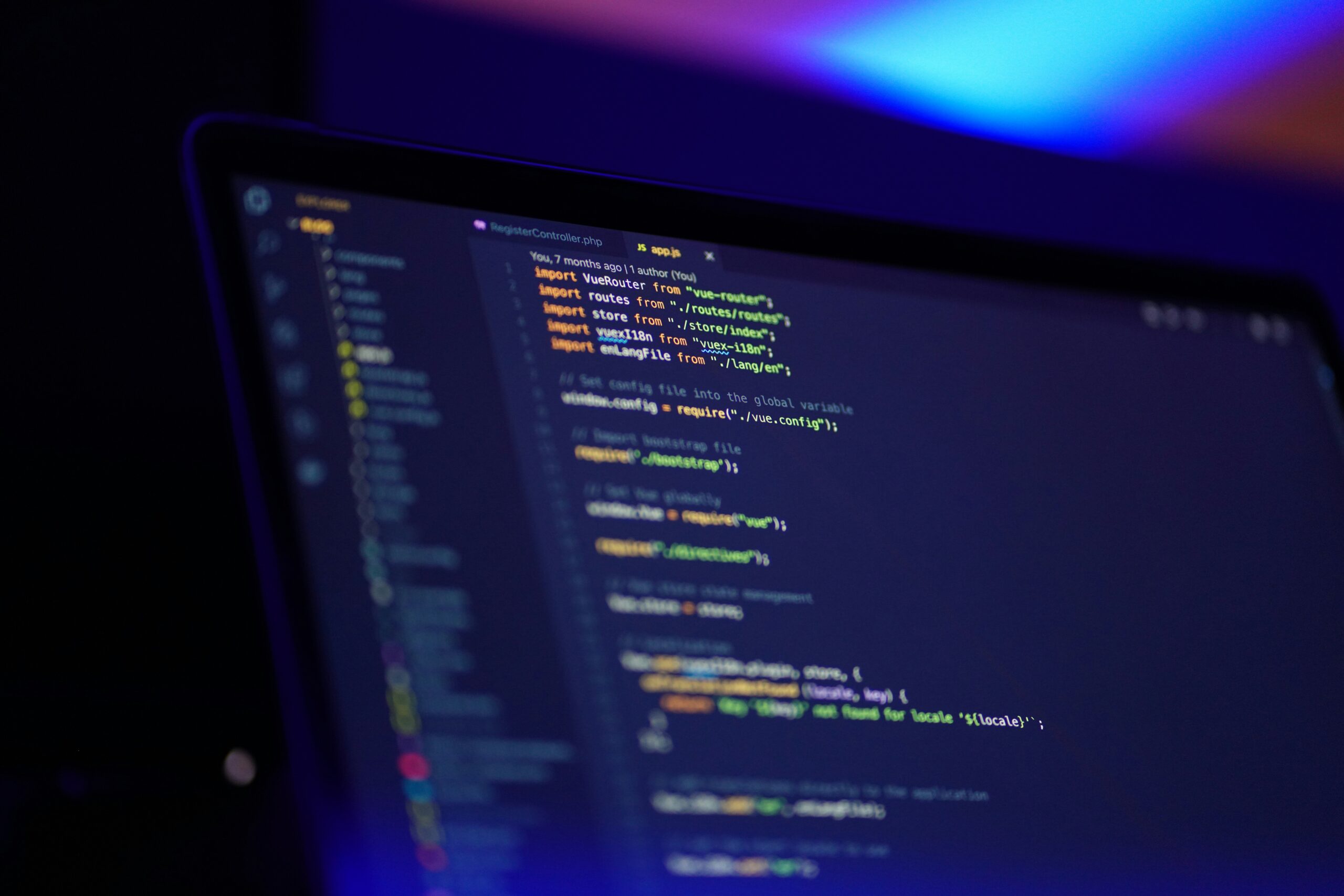
The fundamental principle behind tensor broadcasting is that when performing operations between two tensors of differing shapes, PyTorch will automatically expand the smaller tensor across the dimensions of the larger tensor in accordance with specific broadcasting rules. These rules establish how tensors align in operations based on their shapes, allowing for seamless calculations without manual intervention.
To illustrate, consider two tensors: one of shape (3, 1) and another of shape (3, 4). When performing an addition operation, the smaller tensor (3, 1) is broadcasted across the second tensor (3, 4). Each element of the first tensor is effectively replicated along the second dimension to match the shape of the second tensor. This results in a resulting tensor of shape (3, 4), where each column of the resulting tensor is a copy of the original smaller tensor’s values.
There are specific conditions for broadcasting to occur: the dimensions of the tensors must be compatible. For two tensors to be considered compatible, they must either have the same dimension size or one of them must be of size one. For instance, a tensor of shape (2, 3) can be added to a tensor of shape (1, 3) as the second tensor gets broadcasted to match the dimensions of the first tensor.
Understanding tensors in PyTorch with hands-on examples can significantly enhance one’s ability to manipulate and utilize this powerful feature. By leveraging tensor broadcasting, developers can write more efficient and cleaner code, resulting in a more streamlined development process when using PyTorch for machine learning tasks.
Moving Tensors Between CPU and GPU
In the realm of deep learning and numerical computations, utilizing hardware accelerators, such as GPUs, can significantly enhance performance. PyTorch provides efficient mechanisms for transferring tensor data between the CPU (Central Processing Unit) and GPU (Graphics Processing Unit). This process is crucial for maximizing computation speed, particularly when dealing with large datasets and complex models. Understanding tensors in PyTorch with hands-on examples becomes increasingly essential as practitioners integrate GPUs into their workflows.
To facilitate the movement of tensors, PyTorch offers methods such as .to()
, .cuda()
, and .cpu()
. The .to()
method is versatile, allowing users to specify a device for the tensor, be it a CPU or a specific GPU. For instance, to move a tensor to the GPU, one would use the following command:
tensor = tensor.to('cuda:0')
This command transfers the tensor to the first GPU (if available). Conversely, to return the tensor to the CPU, the method can be utilized in the opposite manner:
tensor = tensor.to('cpu')
The .cuda()
method is more specialized, indicating a straightforward transfer to the default GPU. This method, albeit less flexible than .to()
, is often sufficient for straightforward tensor management.
As an example, if we have a tensor defined as:
tensor = torch.randn(3, 3)
we can transfer it to the GPU using:
tensor = tensor.cuda()
Utilizing GPU for tensor computations not only shortens processing times but also allows deeper models to be trained more effectively. It is essential, however, to monitor the transfer overhead, as moving data between CPU and GPU can introduce latency. As a best practice, it’s recommended to keep tensors on the GPU as long as possible during computation, only transferring them back to the CPU for operations that require CPU access or data output.
Saving and Loading Tensors
Saving and loading tensors is a fundamental aspect of working with PyTorch, particularly in the context of ensuring that machine learning workflows are efficient and reproducible. Tensors, being multi-dimensional arrays, can often occupy substantial amounts of memory, making it crucial to save them for later use without the need to recompute or reinitialize them. PyTorch provides an intuitive approach to tensor persistence through the torch.save()
and torch.load()
functions, which we will explore in this section.
To begin with, the torch.save()
function allows users to save tensors to a file, which can later be retrieved using torch.load()
. This is particularly useful in scenarios where training models takes a considerable amount of time and resources. For instance, after training a model and obtaining a tensor representing the model weights, you can persist these weights to disk as follows:
import torch# Create a tensortensor_data = torch.randn(3, 3)# Save the tensor to a filetorch.save(tensor_data, 'tensor_file.pt')
In this example, we generate a random 3×3 tensor and save it to a file named tensor_file.pt
. When it comes time to load the saved tensor, the process is equally straightforward:
# Load the tensor back into memoryloaded_tensor = torch.load('tensor_file.pt')
After executing this code, loaded_tensor
will contain the same data as tensor_data
. This capability to save and load tensors is not only convenient, but also plays a critical role in scenarios like model evaluation and inference, where you may want to avoid the overhead of reprocessing data. Furthermore, it aids in collaborative workflows where different team members may need to share tensor data without re-running complex computations.
Understanding the mechanisms behind saving and loading tensors in PyTorch can greatly enhance the efficiency of machine learning models, enabling practitioners to focus on refining their algorithms rather than on repetitive data management tasks.
Conclusion and Further Learning Resources
Throughout this blog post, we have explored the foundational concepts of understanding tensors in PyTorch with hands-on examples. Tensors, as the primary data structure in PyTorch, serve a crucial role in developing machine learning models and performing complex mathematical operations. By examining their properties, dimensions, and common operations, we have equipped ourselves with essential knowledge to leverage PyTorch effectively in real-world applications.
One of the primary takeaways from this discussion is the flexibility and efficiency of tensors in managing multi-dimensional data. The significant performance gains offered by PyTorch, especially with GPU acceleration, highlight the importance of mastering tensor manipulation. Furthermore, exploring the various ways to create and transform tensors lays a solid groundwork for more advanced topics, such as implementing neural networks and working with complex datasets.
For those interested in delving deeper into the subject of tensors and PyTorch, several resources can enhance your understanding. The official PyTorch documentation is an invaluable starting point, providing comprehensive guides and practical examples that can broaden your knowledge base. In addition, online platforms like Coursera and edX offer curated courses focused on PyTorch, which cover both introductory and advanced concepts, including tensor operations, deep learning frameworks, and more.
Contributing to community discussions and forums, such as those found on Stack Overflow or the PyTorch discussion boards, can also be beneficial. Engaging with other learners and experts in the field will help reinforce your understanding and provide valuable insights. Lastly, consider exploring books dedicated to PyTorch and machine learning, as they often contain structured examples and practical exercises designed to deepen your grasp of tensors in the context of real-world applications.
- Name: Sumit Singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom