Introduction to Python
Python is a high-level programming language that was created by Guido van Rossum and first released in 1991. Its design philosophy emphasizes code readability, which allows programmers to express concepts in fewer lines of code compared to other languages. This feature, along with its comprehensive standard library, has contributed to its widespread adoption in the tech industry.
One of the defining characteristics of Python is its simplicity and ease of learning. The language’s syntax is clear and straightforward, making it an ideal choice for beginners. Moreover, Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming, which provides flexibility for developers depending on their project requirements. Additionally, the vast ecosystem of Python libraries and frameworks, such as Django for web development and NumPy for data science, expands its functionality and usability across various domains.
In recent years, Python has surged in popularity, consistently ranking among the top programming languages worldwide. This rise can be attributed to its effectiveness in diverse applications, including web development, data analysis, artificial intelligence, and scientific computing. Organizations such as Google, NASA, and Spotify utilize Python due to its versatility, allowing developers to build robust applications efficiently. Furthermore, the strong community support surrounding Python fosters collaboration and resource sharing, making it easier for newcomers to find help and documentation.
Given its robust features and broad applications, understanding the function of Python is essential for anyone looking to enter the tech field or improve their programming skills. The next sections will delve deeper into the specific functions of Python, demonstrating its capabilities and why it remains a preferred choice among developers today.
What is a Function in Python?
A function in Python is a block of reusable code that is designed to perform a specific task. Functions provide a method to organize code into logical segments, thereby enhancing readability and maintainability. When we refer to the function of Python, we recognize its pivotal role in breaking down tasks into smaller, manageable parts. This modular approach allows programmers to write cleaner code and helps in debugging and testing individual components efficiently.
The primary purpose of functions is to encapsulate code that can be called multiple times throughout a program. This reusability not only saves time and effort but also reduces the likelihood of errors. When a function is defined with a specific name, it can be invoked at any point in the code by simply referencing that name, along with the necessary input parameters if applicable. This leads to less redundancy and a clearer structure, as functions enable coders to avoid repeated code blocks. Instead, they can focus on the logic and functionality of their applications.
Moreover, using functions in Python offers several advantages. Functions can take input arguments and return values, which facilitates an interaction between various parts of the program. This encourages a clear separation of logic, where complex processes can be distilled into simple, callable elements, enhancing the overall design. By establishing a common standard for code organization, the function of Python supports collaboration among development teams, allowing multiple programmers to work on different functions concurrently without conflict.
Ultimately, understanding how to define and utilize functions in Python is crucial for anyone looking to develop efficient Python applications. Functions not only streamline projects but also foster a collaborative environment where code can be shared and improved upon over time.
Syntax of Functions in Python
In Python, defining a function is a straightforward process, governed by specific syntax that ensures clarity and readability. The first essential component is the ‘def’ keyword, which stands for ‘define.’ This keyword indicates the start of the function definition, instructing the Python interpreter to recognize that a new function is being created.
Following the ‘def’ keyword, the programmer must provide a unique name for the function. This name should adhere to the naming conventions of Python, which generally means that it should start with a letter or an underscore and can include letters, numbers, and underscores. The function’s name should reflect its purpose, aiding in code readability and maintenance.
Another critical component of the function syntax is the use of parentheses. Within these parentheses, parameters can be defined. Parameters act as variables that can accept values when the function is called. They are optional; a function may also be defined without parameters, serving a specific purpose without requiring input values. It is best practice to provide default values for parameters if applicable, enhancing the function’s versatility.
After the parentheses, there should be a colon. This signifies the start of the function body, which must be indented to define the scope of the function. Inside the body, the programmer can write code that specifies what the function should accomplish. For functions that return a value, the ‘return’ statement is essential, allowing the function to pass back a result when it is called.
As an illustration, a simple function definition in Python might look like the following:
def greet(name):return f"Hello, {name}!"
This function, named ‘greet,’ takes one parameter called ‘name’ and uses the return statement to send back a greeting string. Through this clear syntax, the function of Python facilitates the creation of both simple and complex functionalities within a program.
Types of Functions in Python
The function of Python encompasses a range of different types, which fulfill various programming needs. Among these, built-in functions, user-defined functions, and lambda functions are the most frequently utilized, each offering unique advantages in coding practices.
Built-in functions are pre-defined within Python, formed to carry out a specific task. They can be invoked without the need for any additional definition, making them readily accessible to programmers. Common examples include len()<!-- for computing the length of a list or string,
max()
for determining the highest value in an iterable, and sum()
which computes the total of numerical values. These functions streamline coding by eliminating the necessity for reinventing the wheel for everyday tasks.
User-defined functions, on the other hand, allow programmers to create custom functions tailored to specific requirements. By using the def
keyword followed by the function name and parameters, developers can encapsulate logic into modular components. For instance:
def greet(name):return f"Hello, {name}!"
This user-defined function greet()
takes an individual’s name as input and returns a personalized greeting. Such flexibility in crafting functions aids in code organization, promotes reusability, and simplifies debugging.
Lastly, lambda functions present a compact way to create anonymous functions within Python. Lambda expressions are especially useful when a simple function is needed temporarily for short-term use. They utilize the lambda
keyword, enabling the definition of a function without formally naming it. For example:
multiply = lambda x, y: x * y
In this case, the lambda function multiply
computes the product of two arguments and can be used wherever required. In conclusion, understanding the different types of functions in Python enhances a programmer’s ability to write effective and efficient code, enabling a smooth flow of logic and functionality throughout the development process.
Parameters and Arguments in Functions
The function of Python is built around the concept of functions, which are defined using parameters and arguments. Understanding the distinction between these two concepts is crucial for effective programming. Parameters are the variables that are listed in a function’s definition, acting as placeholders for the values that will be passed to the function. On the other hand, arguments are the actual values assigned to these parameters when a function is called. This distinction forms the foundation for efficient data handling in Python.
To pass data to functions in Python, several methods can be utilized, including positional arguments, keyword arguments, and default parameters. Positional arguments are those that are passed in the order in which they are defined in the function. This means that the first argument corresponds to the first parameter, and so on. For instance, consider a function defined as def greet(name, age):
. When called with greet("Alice", 30)
, “Alice” becomes the value of name
and 30 becomes the value of age
.
Keyword arguments offer greater flexibility by allowing the caller to specify parameters by name, regardless of their order in the function definition. For example, calling greet(age=30, name="Alice")
achieves the same outcome as the previous example, but without being constrained by order. This approach enhances code readability and maintainability.
Additionally, Python allows the use of default parameters, which enables functions to have default values for certain parameters. If no argument is provided for these parameters during the function call, the default value is assigned. A function can be defined as def greet(name="Unknown", age=0):
, allowing it to be called with greet()
, which would utilize default values, or with specific arguments like greet("Alice", 30)
.
Understanding the function of Python in terms of parameters and arguments is essential for writing efficient and effective code. By mastering these concepts, developers can create functions that are both versatile and easy to use.
Return Statement and Its Importance
In Python, the return statement plays a pivotal role in the execution and functionality of functions. Essentially, the return statement is employed to exit a function while passing back an optional value to the caller. This process effectively transfers control from the function back to the point where it was called, allowing further processing of the returned data if needed. The absence of a return statement would lead to the function exiting without providing any resulting values, which could limit its utility.
When a function executes a return statement, it can return various types of data, including numbers, strings, lists, or even other functions. This flexibility makes Python functions exceptionally powerful, as they can encapsulate complex logic and deliver specific outcomes based on inputs. For example, consider a simple function that computes the square of a number:
def square(number):return number * number
In this function, the return statement sends the squared value back to wherever the function was called, allowing further actions to be taken with that result. This mechanism of transferring control and returning values is fundamental in programming, as it promotes modularity and code reusability. By structuring Python functions to return specific outcomes, developers can streamline their code, making it more readable and efficient.
Moreover, the return statement allows functions to be composed, enabling a series of function calls where the output of one function can be directed as input to another. This forms the basis of functional programming paradigms within Python, illustrating how vital the return statement is in utilizing the full potential of the language. In conclusion, understanding the return statement in Python functions not only enhances one’s programming capabilities but also leads to more effective and dynamic code structures.
Scope of Variables in Functions
In Python, the concept of variable scope is crucial for understanding how variables behave within functions. The scope of a variable refers to the context within which it is defined and can be accessed. In the realm of Python, variables can primarily be classified as local or global. Local variables are defined within a function and can only be accessed within that function, essentially being created when the function is called and destroyed once it completes execution. For instance:
def my_function():local_variable = 10return local_variableprint(my_function())# Outputs: 10print(local_variable)# Would raise a NameError
On the other hand, global variables are defined outside of any function and can be accessed from anywhere within the module, including inside functions. However, to modify a global variable within a function, the global
keyword must be used, which allows modification of the global variable rather than creating a local instance. Consider this example:
global_variable = 5def modify_global():global global_variableglobal_variable = 10modify_global()print(global_variable)# Outputs: 10
The lifetime of variables is also influenced by their scope. Local variables exist only during the execution of the function, whereas global variables persist throughout the program’s runtime. It is important to recognize how variable accessibility is affected by scope; this understanding helps avoid common pitfalls like variable shadowing, where a local variable may inadvertently mask a global variable, leading to confusion in code behavior.
Overall, grasping the function of Python regarding variable scope is vital for writing clear and effective code. Understanding local and global variables, their lifetimes, and how their scopes influence accessibility allows developers to utilize Python more effectively in various programming tasks.
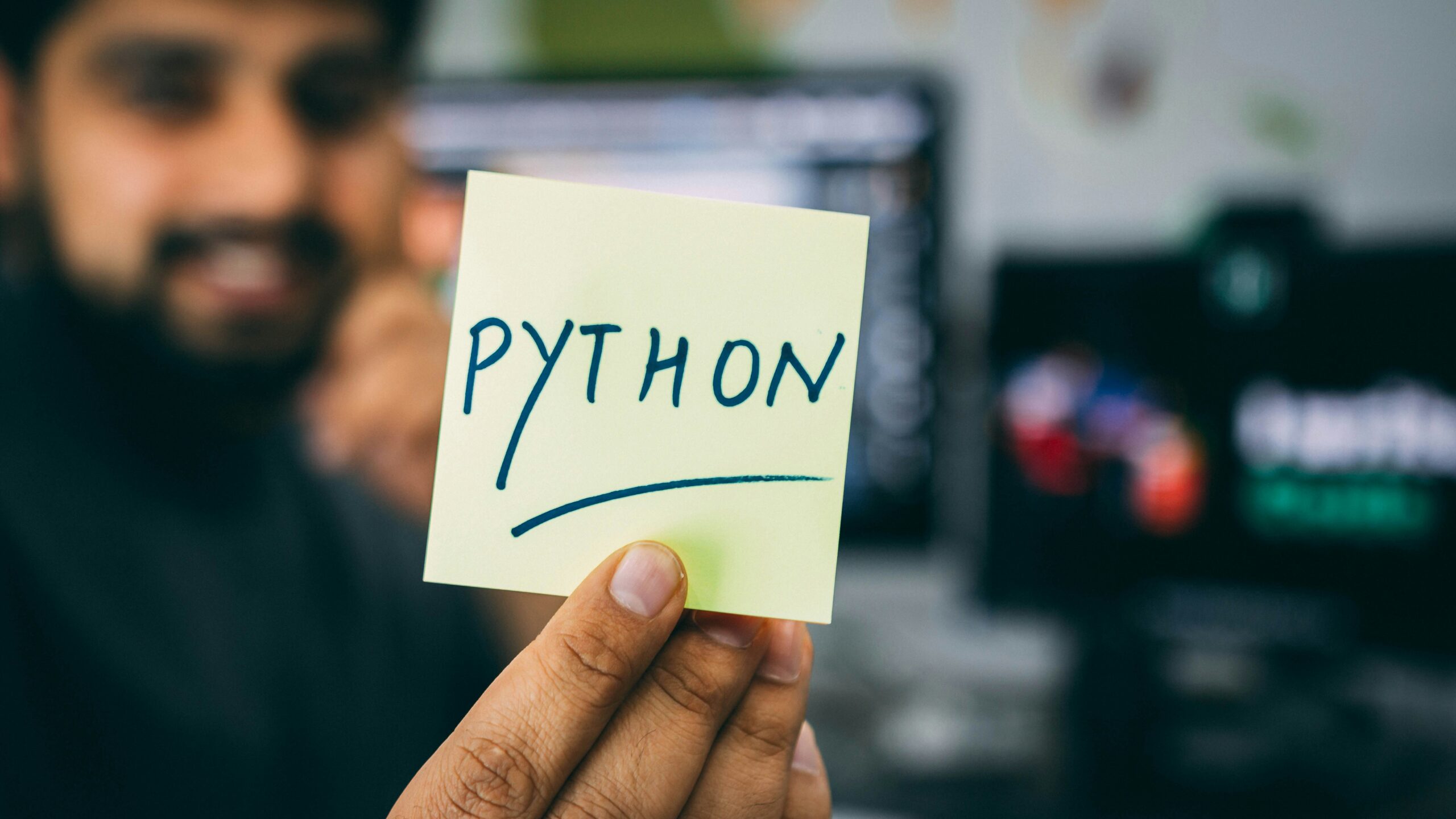
Common Use Cases for Functions in Python
Functions in Python, fundamental building blocks in programming, serve various purposes across different domains. Their versatility becomes evident in several practical applications, particularly in data processing, algorithm implementation, and code organization.
In data processing, functions help streamline operations on datasets. For instance, in a data analysis project, a function can aggregate sales data by region, automate calculations such as average sales, or facilitate data cleaning processes. By encapsulating complex operations into reusable functions, developers enhance the clarity and efficiency of their code, which simplifies both debugging and maintenance. As an example, a function that takes a dataset as input and outputs cleaned data significantly reduces the need for repetitive code.
Algorithm implementation showcases another critical use of functions. Functions allow for the modular approach in coding where each function can represent a distinct algorithm. For example, a sorting algorithm can be implemented in a function, improving readability and organization. Each sorting approach, be it quicksort or bubblesort, can be separated into distinct functions, making it easier to test and optimize each method individually. This separation of concerns ensures that each piece of code has a single responsibility, leading to cleaner and more maintainable systems.
Moreover, functions play a vital role in code organization. A well-structured codebase divides logic into clearly defined functions that can be easily navigated. For instance, in web development, various functions can manage different aspects of a web application, such as handling user input, processing data, and rendering the output. This hierarchical structure not only benefits collaboration, as multiple developers can work on different functions simultaneously, but it also aligns with best practices in software development that advocate for modularity and code reusability.
In conclusion, functions in Python are instrumental across numerous applications, enhancing the programming experience through improved data processing, efficient algorithm implementation, and superior code organization. Their significance is evident in fostering cleaner, more organized, and efficient code overall.
Conclusion: The Role of Functions in Python Programming
Functions play a crucial role in Python programming, serving as a foundational element that promotes modularity and code reusability. Throughout this guide, we have explored several aspects of the function of Python, highlighting their significance in efficient coding practices. By encapsulating operations within functions, Python developers can create cleaner and more maintainable code, which facilitates debugging and enhances collaboration among programmers.
One of the primary advantages of using functions is that they allow developers to break down complex problems into smaller, manageable components. This modular approach not only makes it easier to understand the logic of the code but also enables better organization of tasks. By defining specific functionalities within functions, programmers can call these functions whenever needed, reducing redundancy and improving the readability of the overall program.
Moreover, the versatility of functions in Python extends to the rich array of built-in functions and the ability to create user-defined functions tailored to specific needs. This flexibility empowers developers to leverage pre-existing functionalities and enhance their code with custom logic, fostering an adaptable coding environment. Functions also support various programming paradigms, including procedural and object-oriented programming, which further illustrates their importance in the programming landscape.
As we conclude this exploration of the function of Python, it is essential for aspiring coders to practice creating and utilizing functions within their programming endeavors. By doing so, they will not only improve their coding skills but also contribute to the efficiency and functionality of their projects. Emphasizing the use of functions will lead to more robust and error-free code, positioning developers for success in the dynamic world of programming.
Read more blogs https://eepl.me/blogs/
For More Information and Updates, Connect With Us
- Name: Sumit Singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom