Introduction to Tuples
In the realm of Python programming, tuples are an essential data structure that offers a variety of advantages. A tuple is an immutable sequence type, which means once it is created, its contents cannot be changed. This essential characteristic distinguishes tuples from lists, which are mutable and allow for modification of their elements. The immutable nature of tuples adds a layer of data integrity that can be beneficial in certain scenarios where a fixed collection of items is needed.
Structurally, a tuple is defined by enclosing a series of values within parentheses, separated by commas. For example, a simple tuple can be created as follows: my_tuple = (1, 2, 3)
. This structure not only provides clarity but also ensures that the items within the tuple maintain their order. Another critical aspect of tuples is their ability to hold heterogeneous data types, meaning that a single tuple can contain integers, strings, and even other tuples. This versatility makes tuples a favored choice for storing related pieces of information that are accessed as a single entity.
Among the key features of tuples is their efficiency in data retrieval. Due to their immutability, tuples are typically faster to access compared to lists. This performance advantage can be especially relevant when working with large collections of data, as they consume less memory. Furthermore, tuples can be used as keys in dictionaries, another area where lists fall short due to their mutability. The combination of these properties makes them an integral tool within the broader Python programming landscape. In summary, understanding tuples is crucial for leveraging their advantages while programming in Python.
Creating Tuples
In Python, a tuple is a versatile and immutable data structure that can be utilized for various purposes. The creation of tuples is straightforward and can be achieved through several methods, allowing flexibility in how data is organized. The most common way to create a tuple is by using parentheses. For example, a simple tuple containing multiple elements can be defined as follows:
my_tuple = (1, 2, 3, 4)
. In this example, the tuple my_tuple
contains four integer elements. Python also supports single-element tuples, which require a trailing comma to distinguish them from regular parentheses. A single-element tuple can be created like this: single_tuple = (1,)
.
Alternatively, tuples can be constructed from other iterable data types such as lists. This can be done using the built-in tuple()
function, which takes an iterable as its argument and returns a corresponding tuple. For example, consider the following code snippet:
my_list = [1, 2, 3]
my_tuple_from_list = tuple(my_list)
. Here, my_tuple_from_list
will be a tuple with the same elements as my_list
, resulting in (1, 2, 3)
.
It is essential to recognize that tuples are immutable, meaning once created, their contents cannot be changed. This characteristic can lead to increased performance and security, making tuples a preferred choice in scenarios where data integrity is crucial. Understanding the creation of tuples and the various methods available can enhance your proficiency in Python programming, thus enabling you to utilize this powerful data structure effectively. Each method of creating a tuple provides an avenue for organizing your data in a way that best fits your programming needs.
Accessing Tuple Elements
In Python, tuples are immutable sequences that allow the storage of a collection of items. Accessing elements within a tuple is fundamental for any programming endeavor, as it facilitates data retrieval and manipulation. This section will address the key methods of accessing tuple elements, including indexing, slicing, and iteration.
To access an individual item within a tuple, one can utilize indexing. Python supports zero-based indexing, which means the first element can be accessed using the index position 0. For example, if we define a tuple as my_tuple = (10, 20, 30, 40)
, we retrieve the first element simply by referencing my_tuple[0]
, resulting in an output of 10. Indexing can also be employed to access elements from the end of the tuple using negative indices. Therefore, my_tuple[-1]
would yield the last element, which is 40 in this case.
In addition to indexing, slicing is another robust method for accessing portions of a tuple. Slicing allows users to obtain a range of elements by specifying a start and stop index. For instance, my_tuple[1:3]
will return a new tuple including the second and third elements, hence producing (20, 30)
. It is crucial to note that the stop index is exclusive, thus including elements from the start index up to but not including the stop index.
Lastly, iterating through tuples can be achieved using loops, such as a for
loop, which enables users to process each element in the tuple sequentially. For example:
for item in my_tuple:print(item)
This simple loop will print each element within the tuple, thus illustrating an effective way to access and utilize tuple data. By mastering these techniques, developers can efficiently manipulate and retrieve information stored in tuples, enhancing their overall programming capabilities.
Immutability of Tuples
In the realm of Python programming, understanding the concept of immutability is fundamental, particularly when it comes to data structures such as tuples. A tuple is defined as an ordered collection of elements which, once defined, cannot be altered or modified. This characteristic is primarily what sets tuples apart from lists, which are mutable and allow for changes in their respective elements. Immutability in tuples ensures that once a tuple is created, its contents remain intact throughout its lifetime.
The implications of immutability in tuples are significant in terms of data integrity and performance. For instance, since tuples cannot be altered after creation, they can be used as keys in dictionaries, unlike lists which, due to their mutable nature, cannot guarantee a stable hashing mechanism. This makes tuples particularly advantageous for cases where a constant data structure is required, allowing for predictable behavior in data storage and retrieval operations.
To illustrate this further, let’s consider a simple example. If a tuple is defined as my_tuple = (1, 2, 3)
, attempting to change an element, such as my_tuple[1] = 4
, will result in a TypeError
. Conversely, with a list, the same operation would execute without error: my_list = [1, 2, 3]; my_list[1] = 4
successfully changes the second element to 4. This fundamental behavior of tuples being immutable underlines their reliability when dealing with fixed collections of data.
In conclusion, the immutability of tuples in Python presents both advantages and limitations. This property not only bolsters data integrity but also provides performance benefits, making tuples a preferred choice for certain programming scenarios over their mutable counterparts, such as lists.
Tuple Operations
Tuples in Python are immutable sequences, which means that once a tuple is created, its contents cannot be modified. However, numerous operations can be performed on tuples that facilitate working with this data structure effectively. One common operation is concatenation, which allows you to combine multiple tuples into one. For example, given two tuples, tuple1 = (1, 2, 3)
and tuple2 = (4, 5)
, concatenating them can be accomplished by using the +
operator: result = tuple1 + tuple2
, yielding (1, 2, 3, 4, 5)
.
Another useful operation is repetition, where you can repeat a tuple multiple times to create a new tuple containing repeated elements. For instance, if you have tuple3 = (0, 1)
, using the repetition operator *
allows you to create result = tuple3 * 3
, which results in (0, 1, 0, 1, 0, 1)
. This feature can be particularly beneficial when initializing tuples with default values for use in various applications.
Additionally, membership testing is a valuable operation that lets you check the presence of an element within a tuple. This can be accomplished using the in
keyword. For example, if you have tuple4 = ('a', 'b', 'c')
, you can determine if 'b'
is in tuple4
by performing the test 'b' in tuple4
, which would return True
. Such operations contribute significantly to the usability of tuples in Python programming, facilitating efficient data handling and retrieval.
Tuple Methods
In Python, tuples are immutable sequences that serve various purposes. The built-in methods associated with tuples provide functionality that enhances their usability in programming. Two of the primary tuple methods are count()
and index()
. Understanding these methods is essential for efficiently handling data stored within tuples.
The count()
method returns the number of occurrences of a specified value within a tuple. For instance, if a tuple contains multiple instances of a particular element, the count()
method can quickly ascertain how many times that element appears. Here’s an example: consider the tuple numbers = (1, 2, 2, 3, 4, 2)
. By executing numbers.count(2)
, the output will be 3
, indicating that the number ‘2’ appears three times. Such functionality is beneficial in scenarios where data analysis—such as frequency distribution—is required.
Another useful method is index()
, which finds the position of the first occurrence of a specified value. This method is particularly useful when one needs to locate the index of a specific element in a tuple. For example, if we use the tuple fruits = ('apple', 'banana', 'cherry', 'banana')
, calling fruits.index('banana')
would return 1
as the index of its first appearance. This can be particularly advantageous when managing databases or datasets where one needs to quickly locate the position of data points.
In practicing Python, it is paramount to leverage these methods fully, as they can significantly enhance the efficiency of your code and the clarity of your data manipulation. Understanding tuple methods such as count()
and index()
ultimately contributes to mastering the data structures in Python programming.
Unpacking Tuples
In Python, tuples are immutable sequences, meaning that once they are created, their contents cannot be modified. One of the notable features of tuples is their ability to be unpacked, which allows for a more efficient and readable way to assign values. Tuple unpacking refers to the process of assigning the elements of a tuple directly to a set of variables in a single statement, streamlining the code and making it cleaner.
To illustrate this concept, consider the following example. Suppose we have a tuple consisting of three elements:
coordinates = (10, 20, 30)
By employing tuple unpacking, we can directly assign these values to multiple variables:
x, y, z = coordinates
After executing this statement, the variables x
, y
, and z
will hold the values 10
, 20
, and 30
, respectively. This method not only enhances code clarity but also reduces the chances of errors that might arise from repeatedly indexing into the tuple.
Another advantage of tuple unpacking is its applicability within loop structures. For instance, when iterating over a list of tuples, it permits simultaneous assignment of values directly within the loop:
points = [(1, 2), (3, 4), (5, 6)]for x, y in points:print(f'X: {x}, Y: {y}')
This usage exemplifies how tuples can streamline the code further, allowing for cleaner, more readable logic. By utilizing tuple unpacking, programmers can maintain conciseness while benefiting from Python’s inherent capabilities.
Ultimately, mastering tuple unpacking provides significant advantages, improving code efficiency and maintainability, while also adhering to Python’s principles of simplicity and clarity.
Tuples vs. Lists: When to Use Which
In Python, understanding the distinctions between tuples and lists is crucial for making informed programming decisions. Both data structures serve distinct purposes and offer unique advantages; however, their implementations and characteristics differ significantly.
Tuples are immutable sequences, meaning once they are created, their contents cannot be altered. This immutability provides benefits in scenarios where a fixed collection of items is required. For instance, tuples are often employed to represent a record of information, such as coordinates in a 2D space or database records, where the integrity of the data must be maintained. Furthermore, because of their immutable nature, tuples can be used as keys in dictionaries, which is not possible with lists.
On the other hand, lists are mutable, allowing for changes to be made after their creation. This flexibility is particularly useful when data needs to be modified, such as adding, removing, or updating elements. Lists are typically chosen for tasks that require frequent modifications, like iterating over a collection of items or managing dynamic datasets. In performance terms, lists may be slightly slower than tuples due to their mutability; however, the difference is often negligible unless the code is critically performance-intensive.
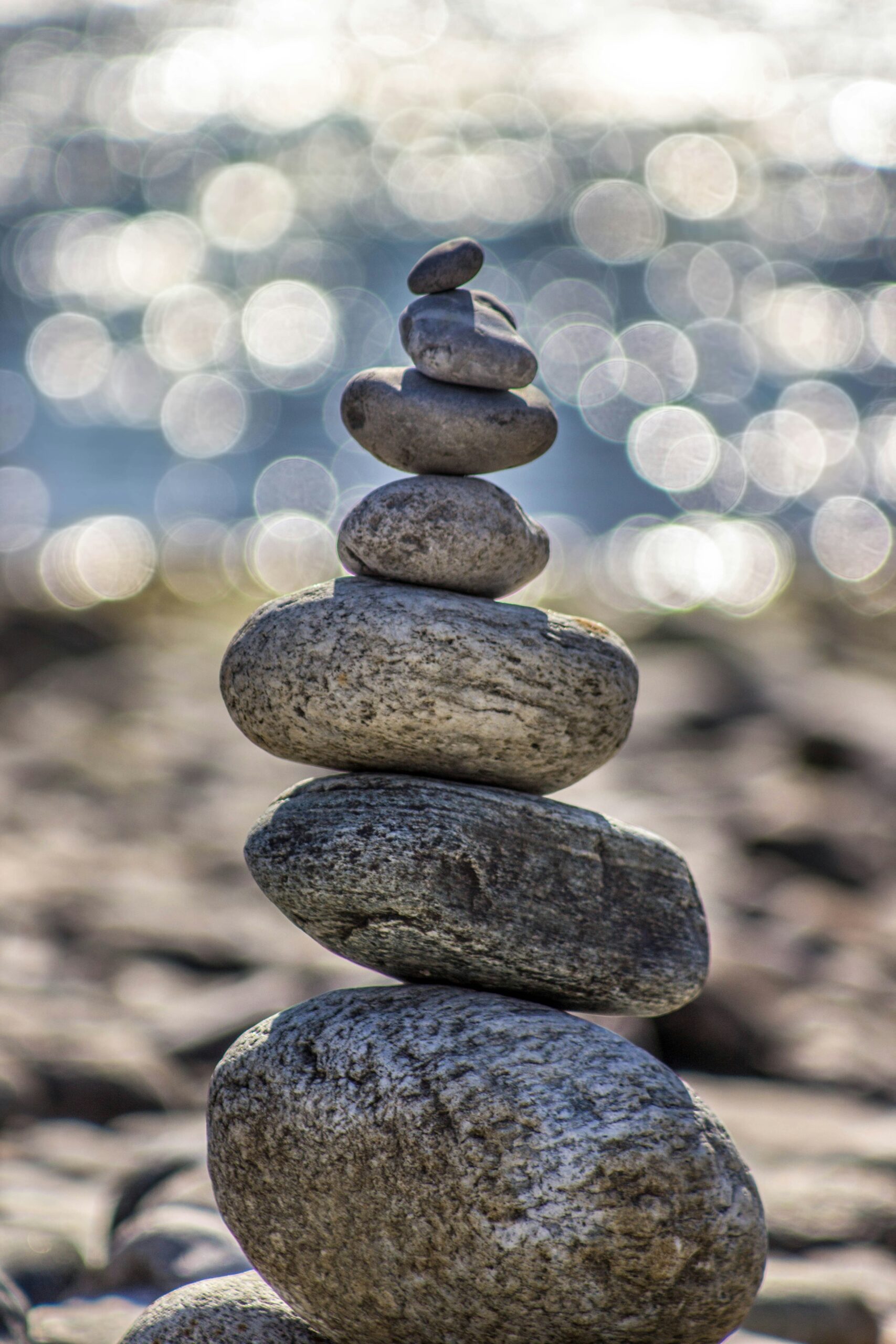
When it comes to memory usage, tuples are generally more memory-efficient than lists. This is because tuples have a smaller memory footprint, as they do not store the overhead associated with modifiable elements. This advantage, combined with their immutability, makes tuples an excellent choice for fixed or read-only datasets, enhancing performance in situations where necessary.
In conclusion, selecting between tuples and lists in Python ultimately depends on the specific requirements of your application. Tuples offer immutability and efficiency, making them suitable for fixed data collections, while lists provide the flexibility and ease of modification beneficial for dynamic data management.
Practical Applications of Tuples in Python
Tuples in Python have garnered attention not only for their simplicity but also for their versatility across various programming tasks. One major application of tuples is in data manipulation, where they serve as a lightweight alternative to lists for storing collections of items. For instance, when storing a set of coordinates, a tuple (x, y) can be utilized, reflecting the unchanging nature of a spatial point while offering a straightforward data structure for access and iteration.
Another practical application of tuples is in returning multiple values from a function. In Python, functions can return a single object, but this object can be a tuple containing several values. For example, a function might compute a person’s age and year of birth, returning both as a tuple (age, year_of_birth). This method not only promotes clean and maintainable code but also enhances the function’s return context, thereby making the output more informative while minimizing the overhead associated with creating a class or a more complex data structure.
Furthermore, tuples are often utilized as keys in dictionaries due to their immutable nature. When the need arises to combine several elements into a unique identifiable key, tuples offer a practical solution. For instance, when tracking the attendance of students in various classes, leveraging a tuple (student_id, class_id) as a key allows for an efficient storage mechanism that maintains the association without the risk of modification. This ability to store multi-dimensional indices in a dictionary further enhances data access and manipulation efficiencies.
In conclusion, the practical applications of tuples in Python are vast and varied. Their immutability, ability to return multiple values from functions, and capacity to act as dictionary keys underscore the benefits of integrating tuples into programming projects. As developers strive for efficiency and clearer code, embracing tuples can lead to more effective and organized data handling solutions.
Read more blogs https://eepl.me/blogs/
For More Information and Updates, Connect With Us
- Name: Sumit Singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom