Introduction to Servlets
Servlets are server-side components that form a fundamental part of Java-based web applications. They are Java classes that operate on a server and respond to client requests, most commonly those originating from web browsers. Their primary purpose is to generate dynamic content, handling multiple types of requests effectively, and managing the interaction between clients and servers. Servlets utilize the capabilities of the Java EE framework, which allows developers to build robust, scalable, and maintainable web applications.
In the Java EE architecture, servlets function as part of the web tier, where they interact with both the client layer and the business logic layer. When a user sends a request to a web application, the web server routes this request to the appropriate servlet. The servlet processes the request by executing the necessary business logic and may interact with databases or other enterprise resources. Following the processing, the servlet generates a response, typically in the form of HTML or other web-readable content, which is then sent back to the client. This request-response cycle is essential in developing interactive web applications.
One of the key advantages of using servlets is their ability to maintain a state across multiple requests by utilizing sessions. This capability is crucial in web applications where user interaction needs to be tracked, such as in e-commerce sites. Moreover, servlets support various protocols, including HTTP, making them versatile in handling different types of web services. Their use in conjunction with other Java technologies, like JSP (JavaServer Pages) and frameworks such as Spring MVC, further enhances their functionality and integration within Java EE applications. Overall, servlets are indispensable in the realm of Java web development, establishing a strong foundation for creating dynamic web applications.
Setting Up the Development Environment
To embark on servlet programming, establishing a robust development environment is essential. The first step involves the installation of the Java Development Kit (JDK). The JDK is a pivotal software development kit that provides tools necessary for developing Java applications, which include the Java Runtime Environment (JRE), Java compiler, and essential Java libraries. The version of JDK should ideally be the latest stable release compatible with your operating system. Once downloaded, follow the installation instructions specific to your platform. Ensure that the JDK’s bin directory is added to your system’s PATH variable, allowing the system to recognize Java commands from any command line interface.
Following the JDK installation, selecting a suitable Integrated Development Environment (IDE) is crucial. Popular choices for servlet programming include Eclipse and IntelliJ IDEA. Both IDEs provide robust support for Java and servlet development, including features such as code completion, debugging tools, and server integration capabilities. To install an IDE, download the installer from the official website, run it, and follow the setup wizard. Once installed, configure the IDE to link with the JDK you previously set up. This allows the IDE to compile and execute Java applications seamlessly.
The next step is to set up a servlet container, which is essential for deploying and running servlets. Apache Tomcat is a widely-used servlet container that provides a robust environment for Java server-side applications. To install Tomcat, download the latest version from the Apache Tomcat website. After extracting the files, configure the server by modifying the ‘server.xml’ file if necessary. To ensure everything is set up correctly, you can deploy a simple servlet by placing the servlet’s compiled class files in the appropriate directory within the Tomcat structure and accessing it via a web browser. Completing these steps establishes a functional development environment for servlet programming, setting the foundation for further exploration into Java web application development.
Understanding the Servlet Lifecycle
The servlet lifecycle is a critical aspect of servlet programming in Java, as it delineates the various stages through which a servlet navigates from its inception to its eventual destruction. This lifecycle consists of three primary phases: initialization, request handling, and cleanup, each of which is managed through specific methods provided by the Servlet API.
The first stage, initialization, is primarily handled by the init()
method. When a servlet is first loaded into memory, the servlet container invokes this method to perform any startup activities. This may include establishing database connections, reading configuration files, or allocating resources. It is important that this method is implemented to efficiently set up the servlet environment, as it is called only once during the servlet’s lifecycle. Consequently, developers should ensure resource management to enhance performance and responsiveness.
Following initialization, the servlet enters the request handling phase. The container invokes the service()
method for every client request that targets the servlet. This method is responsible for processing the incoming request and generating a corresponding response. The service()
method operates by delegating requests to specific handler methods such as doGet()
or doPost()
, based on the HTTP method used by the client. It is within this phase that the servlet effectively communicates with clients and handles multiple requests simultaneously, utilizing the thread-safe capabilities of Java servlets.
Finally, the lifecycle concludes with cleanup, which is managed by the destroy()
method. This method is invoked when the servlet is being taken out of service, typically during server shutdown or when the servlet is unloaded. It allows servlets to release resources such as closing database connections or flushing log files, ensuring that any remaining tasks are completed. Understanding these lifecycle stages is essential for developers to effectively manage resources and optimize the performance of Java web applications.
Creating Your First Servlet
To begin with servlet programming in Java, it is essential to understand the basic structure and components needed to create your first servlet. Servlets are Java classes that handle HTTP requests and generate responses. The first step in creating a servlet is to set up your Java environment and ensure you have a web server that supports servlets, such as Apache Tomcat.
Next, you will need to create a new Java class that extends the HttpServlet
class. This inheritance allows your servlet to handle various HTTP methods such as GET and POST. Here’s a simple structure for your servlet:
import java.io.IOException;import javax.servlet.ServletException;import javax.servlet.http.HttpServlet;import javax.servlet.http.HttpServletRequest;import javax.servlet.http.HttpServletResponse;public class MyFirstServlet extends HttpServlet {protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {response.setContentType("text/html");response.getWriter().println("<h1>Hello, World!</h1>");}}
In this example, the doGet
method is overridden to handle GET requests. The method takes two parameters: HttpServletRequest
, which contains the client request, and HttpServletResponse
, which is used to formulate the response back to the client. Setting the content type, our servlet returns a simple HTML message.
After defining the servlet, the next step is to configure it in the web.xml
file of your web application. This configuration tells the server how to map requests to your servlet. Here is a sample entry:
<servlet><servlet-name>MyFirstServlet</servlet-name><servlet-class>com.example.MyFirstServlet</servlet-class></servlet><servlet-mapping><servlet-name>MyFirstServlet</servlet-name><url-pattern>/hello</url-pattern></servlet-mapping>
With these steps, deploying your servlet will allow you to handle requests and respond accordingly. As you become more familiar with servlet programming, you will discover various ways to optimize your code and handle different types of requests more efficiently. Testing different scenarios will also help you avoid common pitfalls and solidify your understanding of how servlets operate.
Working with HTTP Methods
Servlets play a pivotal role in managing HTTP requests in Java web applications. When it comes to handling requests, servlets can interpret various HTTP methods, including GET, POST, PUT, and DELETE. Each of these methods serves a distinct purpose and has its own set of implications that developers should understand to use effectively in servlet programming.
The GET method is predominantly used for data retrieval. When a client sends a GET request to a servlet, it typically expects a response with resource data, such as HTML pages or JSON objects. GET requests can also include parameters in the URL, which servlet can utilize to tailor the response accordingly. However, it is important to note that GET requests should not modify server state since they are meant to be idempotent.
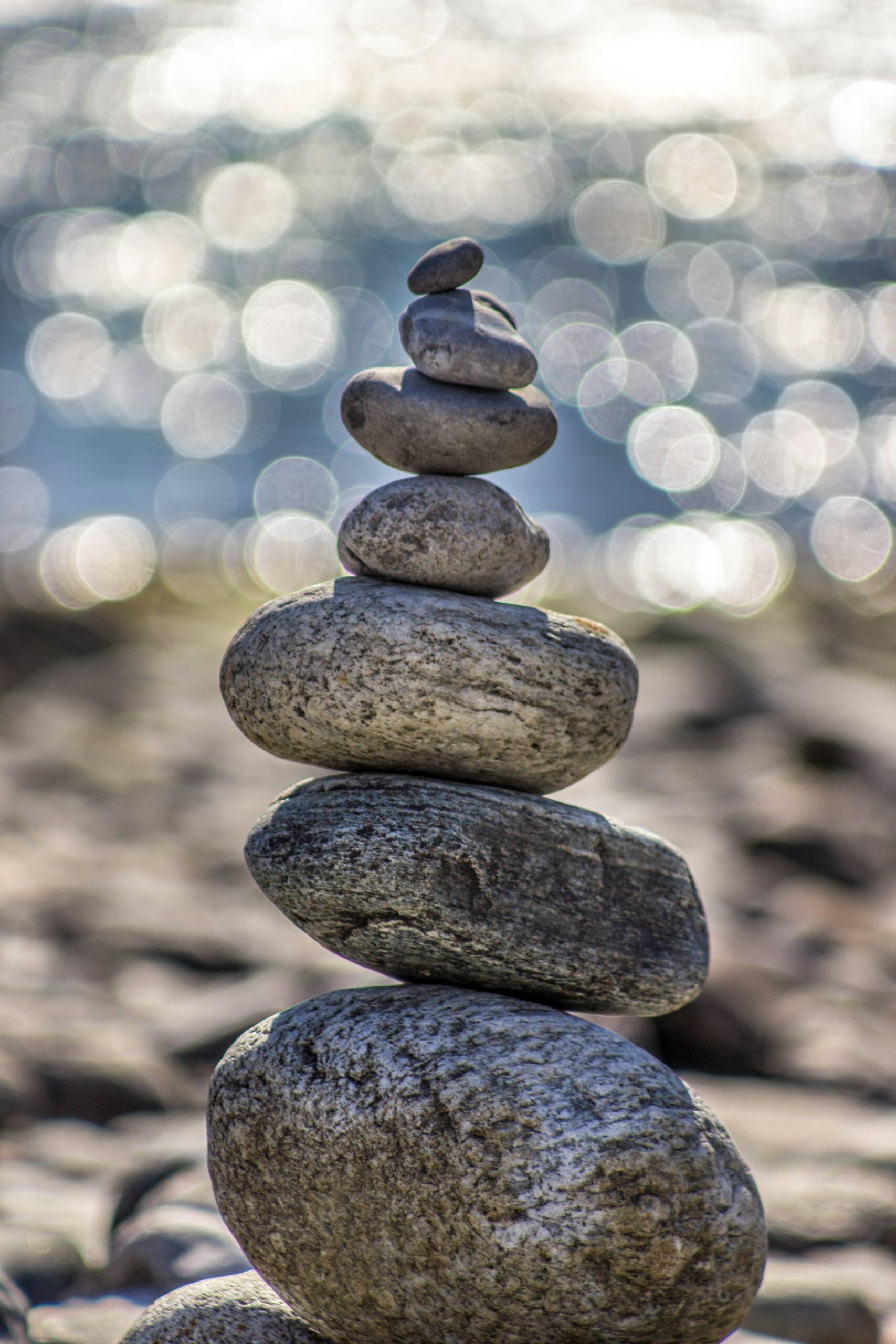
In contrast, the POST method is designed for submitting data to the server, often in the context of form submissions. When a form is submitted, the servlet typically captures this data through the request object. This method allows for a more extensive amount of data to be sent, including complex objects. The handling of POST requests in servlets can involve additional processing like input validation or data saving to a database.
The PUT method is used to update existing resources or create new ones on the server. Servlets handle PUT requests by taking the payload from the request and applying it to the designated resource. Conversely, the DELETE method serves to remove existing resources. In servlet use, it is essential to confirm that the operation is authorized to maintain security and integrity of application data.
Incorporating these HTTP methods appropriately allows servlet-based applications to offer dynamic functionality, such as RESTful services, enhancing user experience through efficient data retrieval, and manipulation processes.
Managing Session in Servlets
In the realm of web applications, managing user sessions is crucial for ensuring a seamless experience across multiple requests. Java Servlets provide the HttpSession interface, which allows developers to handle user data effectively. A session is created when a user first interacts with a servlet, and it is typically initiated through the request.getSession()
method. This method retrieves the existing session associated with the user or creates a new one if none exists. Utilizing this approach permits the application to track user-specific information, thereby enhancing the application’s personalization capabilities.
Once a session is established, developers can store user attributes conveniently using the setAttribute(String name, Object value)
method. This allows a servlet to keep track of relevant information such as user preferences, login status, or shopping cart details. It is important to choose attribute names carefully to avoid conflicts and ensure readability. Moreover, these attributes can be retrieved during subsequent requests using getAttribute(String name)
, fostering a smooth user experience as they revisit various pages within the application.
Session management also involves attention to security and performance. For instance, setting a session timeout through the setMaxInactiveInterval(int interval)
method helps prevent unauthorized access by limiting the duration of a session. Once the timeout period elapses without any activity, the session becomes invalidated. It is essential to communicate this timeout policy in the user interface so that users are aware of potential logouts.
Finally, it is crucial to properly invalidate sessions to free up resources and prevent memory leaks. This can be achieved using the invalidate()
method on the HttpSession object. By following these best practices for session management, servlet developers can ensure not only the integrity of user data but also an enhanced overall experience in web applications.
Servlet Configuration and Deployment
Servlet configuration and deployment are critical processes in Java web application development. These processes allow developers to define how servlets function and interact within a web environment. The configuration of servlets typically occurs through the web.xml
deployment descriptor or by using annotations within the servlet code itself. Understanding these methods is essential for effective servlet management.
To begin with, the web.xml
file acts as a central configuration point for a Java web application. Within this descriptor, servlet mapping can be specified, which outlines the URL patterns associated with specific servlets. By defining a URL pattern, developers can dictate how web requests are routed to their corresponding servlets. For instance, a servlet mapped to the URL pattern /myServlet
will respond whenever a request is made to that endpoint. This mapping process is not only crucial for functionality but also for organizing the application’s structure.
Furthermore, developers can configure servlet parameters within the web.xml
file. These parameters can include initialization values that the servlet will use upon instantiation. By specifying parameters, developers enhance the servlet’s functionality and customize its behavior without altering the servlet’s code. Annotations such as @WebServlet
provide a modern alternative, allowing configuration to be embedded directly within the servlet class. This approach reduces the need for extensive XML configuration, streamlining the development process.
Understanding the application structure is also vital when deploying servlets on a web server. A typical Java web application follows a specific directory layout, which includes locations for classes, libraries, and the web.xml
file itself. When deploying, developers must ensure that these components are appropriately arranged within a .war file to facilitate server-side execution. Following proper deployment procedures helps ensure that servlets operate as intended in a production environment.
Error Handling and Exception Management
In servlet programming, effective error handling and exception management are fundamental to creating robust web applications. When developing servlets, developers must anticipate potential errors that may arise during request processing, which ensures that users can navigate the application smoothly, even when issues occur. One common approach to managing errors is the use of dedicated error pages. By configuring web.xml, developers can specify specific error pages that will be displayed when particular exceptions are thrown or when HTTP errors occur, guiding users gracefully back to appropriate content without exposing them to raw error messages.
Another critical aspect of error management in servlets is logging exceptions. Logging provides valuable insights into application performance and helps track issues that may affect user experience. Java provides multiple logging frameworks such as Log4j or SLF4J that can be easily integrated into servlets to record exception details. This documentation aids developers in diagnosing and resolving problems more effectively. Furthermore, it acts as a reference point for understanding how the application behaves under various conditions, which is invaluable for debugging and performance tuning.
Implementing try-catch blocks is another fundamental practice in managing exceptions within servlet code. By wrapping potentially problematic sections of code within a try-catch construct, developers can catch exceptions and respond accordingly without crashing the application. This method allows for custom handling of different types of exceptions, providing tailored responses based on the nature of the error. For example, database connection issues can trigger specific recovery actions, while client-side input errors can lead to form validation messages being displayed. Together, these strategies contribute to a more resilient servlet application that accommodates users effectively, enhancing overall user satisfaction.
Best Practices and Common Pitfalls
Effective servlet programming requires adherence to established best practices to enhance performance, maintainability, and reliability of web applications. One of the key practices is optimizing resource management. Developers should ensure that they properly manage resources such as database connections and file streams to prevent memory leaks and resource exhaustion. For example, using connection pools can significantly improve the efficiency of database interactions and minimize the connection overhead inherent in servlet operations.
Another important practice is to maintain clean code organization. Structuring servlets and separating business logic from presentation layers helps in keeping the codebase manageable. Utilizing the Model-View-Controller (MVC) pattern is highly recommended for this purpose, as it aids in developing a system that is easier to maintain and extend. By compartmentalizing functionality, developers can also facilitate unit testing, which is crucial for ensuring the reliability of the code.
Performance optimization is another vital aspect when using servlets. Techniques such as caching frequently accessed data can reduce the load on the server and improve response times. Additionally, developers should strive to minimize processing time within the service methods of servlets to enhance user experience. Using asynchronous processing can be beneficial to offload lengthy tasks and prevent blocking of request threads.
Despite having solid practices, developers may encounter common pitfalls while working with servlets. One prevalent error is neglecting to handle exceptions effectively, which can lead to application crashes. Incorporating robust error handling strategies is essential to ensure that the application remains functional even in the event of unexpected issues. Another common mistake is failing to validate user input adequately, which can expose applications to security vulnerabilities.
By following these best practices and being aware of potential pitfalls, developers can create servlet-based applications that are efficient, reliable, and maintainable. Continuous learning and improvement in these areas are crucial for any professional working with servlet technology.
Read more blogs https://eepl.me/blogs/
For More Information and Updates, Connect With Us
- Name: Sumit Singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom