Introduction to Authentication and JWT
Authentication is a critical aspect of web applications, as it serves to verify the identity of users accessing the system. In an era where cybersecurity threats continue to escalate, the need for robust authentication mechanisms is paramount. Implementing effective strategies enhances the protection of sensitive data and builds trust with users. Among these methods, JSON Web Tokens (JWT) have emerged as a popular solution for secure authentication processes in modern applications.
A JSON Web Token is a compact, URL-safe means of representing claims to be transferred between two parties. It consists of three primary components: a header, a payload, and a signature. The header typically indicates the type of token and the signing algorithm being used. The payload contains the claims, which are the statements about an entity (typically, the user) and any additional data. Finally, the signature is created by combining the encoded header, payload, and a secret key. This ensures the integrity of the token and guarantees that it has not been tampered with during transmission.
JWT stands apart from traditional authentication methods such as session-based authentication, where a server retains user sessions. In contrast, JWT adopts a stateless approach, where the server does not store user sessions. This enhances scalability as there is no need for session storage across distributed servers. Additionally, JWT’s compatibility with various platforms and its ability to facilitate role-based authentication further streamline how access tokens are managed.
Understanding the benefits of authentication with JWT allows developers to create secure and scalable login systems effectively. This is particularly relevant as security trends highlight the importance of implementing integration with major APIs such as Google, GitHub, and Facebook, which require refined authentication protocols. Implementing effective authentication with JWT is not just a trend; rather, it is an essential practice in safeguarding modern web applications.
How JWT Works: The Architecture
JSON Web Tokens (JWT) have gained significant traction in the realm of authentication, providing a secure and efficient method for transmitting information. At its core, a JWT is composed of three main components: Header, Payload, and Signature, each playing a pivotal role in ensuring security and integrity. The Header typically specifies the signing algorithm used, providing insight into how the token should be processed. Common choices for signing algorithms include HMAC SHA256 and RSA.
The next component, the Payload, contains the actual claims. These claims can be of various types, including registered claims, public claims, and private claims. For instance, registered claims can include important information such as user roles, expiration times, and unique identifiers. This secure encapsulation of user data is vital, particularly in security trends such as role-based authentication, where user roles dictate access to resources.
Finally, the Signature provides the layer of security that ensures the token has not been tampered with. To create a Signature, the encoded Header and Payload are combined and signed using a secret key or a public/private key pair when working with asymmetric algorithms. This process guarantees that the token’s information remains intact during transmission over HTTP headers.
Encoding a JWT involves converting the Header and Payload into a base64url encoded string, which is then concatenated with the Signature. The resulting token offers a compact representation that can be securely transmitted in web applications. Decoding acts as the reverse process, allowing servers to verify the Signature, extract the claims from the Payload, and authenticate the user seamlessly. In security trends focusing on building secure, scalable login systems, understanding the intricacies of JWT architecture facilitates robust authentication and can lead to effective integration with APIs, such as those provided by Google, GitHub, and Facebook.
Benefits of Using JWT for Authentication
JSON Web Tokens (JWT) offer a range of benefits that make them an attractive option for implementing authentication in modern web applications. Primarily, the statelessness of JWT allows for the storage of user session information on the client side, as opposed to maintaining a session on the server. This means that once a user is authenticated, the server does not need to hold any session data. Instead, the token itself carries the necessary data, significantly enhancing the scalability of systems since reduced server load enables easier management of high-traffic scenarios.
Another key advantage of JWT is their flexibility. With the ability to include various claims, developers can customize tokens to fit specific application requirements. This is particularly useful when integrating with third-party services, such as Google, GitHub, and Facebook APIs, allowing for seamless authentication when users opt to log in using their existing credentials from these platforms. Such integration adds both convenience and security to the user experience.
When comparing JWT to traditional session-based authentication methods, a notable difference is the complexity of session management. Traditional approaches necessitate a centralized database for session storage, leading to challenges around scalability and performance during peak times. Conversely, JWT utilizes a decentralized approach, enabling each client to self-manage their authentication tokens. This not only reduces server-side overhead but also supports the growing trend towards building secure, scalable logging systems, which is a hot topic among developers.
Moreover, JWT supports various algorithms for signing tokens, including HMAC and RSA, which allows developers to implement role-based authentication effectively. By leveraging this capability, applications can define permissions and access levels for different user roles, enhancing overall security. Given these advantages, it becomes clear why many organizations are moving towards authentication methods that utilize JWT for their versatility and robustness in today’s computing environment.
Setting Up a Basic Project for JWT Authentication
To effectively implement authentication with JSON Web Tokens (JWT), it is essential to establish a solid foundation for your project. First, select a programming language and framework that aligns with your project goals. Popular choices include Node.js with Express, Python with Flask or Django, and Ruby on Rails. Each of these frameworks has robust support for implementing security trends, including refresh tokens, access tokens, and role-based authentication.
After determining the framework, the next step is to install the necessary libraries that facilitate JWT authentication. For instance, if you opt for Node.js, you can use the ‘jsonwebtoken’ package to create and verify tokens. In a Python project, libraries such as ‘PyJWT’ serve a similar purpose. These libraries simplify the implementation of secure, scalable login systems, which is a hot topic in web security today.
Once the software libraries are installed, prepare your development environment. This includes setting up a local server and structuring your project files. It is advisable to organize your files with clear directories for routes, models, and controllers to enhance maintainability. Depending on the chosen framework, you may also need to integrate with third-party APIs, such as Google, GitHub, and Facebook, for additional authentication options.
Ensure you have a configuration file that manages your environment variables, like secret keys for signing JWTs. This step contributes to enhancing security by keeping sensitive information out of the public codebase. Applying best practices in your setup will not only streamline the development process but also create a robust authentication mechanism.
In summary, setting up a basic project for JWT authentication involves selecting the appropriate programming language and framework, installing the necessary libraries, and preparing an organized coding environment. This structured approach lays the groundwork for implementing effective authentication methods that align with current security trends.
Creating JWT Tokens: A Step-by-Step Guide
Json Web Tokens (JWT) provide a compact and self-contained way for securely transmitting information between parties as a JSON object. The process of creating JWT tokens involves several critical steps that are designed to ensure the generated tokens are secure and efficient. Below, we outline a clear and concise approach to implementing JWT in your applications.
To begin, it is essential to identify the libraries and tools needed for JWT creation. Many programming languages offer robust libraries for JWT handling. For instance, if you are using Node.js, you can utilize the ‘jsonwebtoken’ package. Start by installing this package using npm:
npm install jsonwebtoken
Once the package is installed, you can create a JWT by invoking the `sign` method, which will accept parameters such as the payload, secret key, and options. Here’s a simple code snippet to demonstrate:
const jwt = require('jsonwebtoken');const payload = { userId: 123, role: 'admin' }; // Example payloadconst secretKey = 'your_secret_key'; // Replace with your secret keyconst token = jwt.sign(payload, secretKey, { expiresIn: '1h' }); // Token valid for 1 hourconsole.log(token);
The above snippet generates a JWT encoded with the payload containing user information, such as user ID and role. This token can be utilized for user authentication and role-based authentication, an essential aspect of secure, scalable login systems. It is also crucial to ensure that secret keys are stored securely and never hard-coded into the application code.
Moreover, when creating JWT tokens, consider implementing security trends such as refresh tokens alongside access tokens. This dual-token approach enhances security by limiting the lifespan of access tokens and allowing users to seamlessly obtain new tokens without re-authentication. Additionally, employing integration with popular add-ons, such as Google, GitHub, and Facebook APIs, can streamline authentication processes for users as they can leverage existing accounts to access your services.
By following these steps and adhering to best practices, developers can successfully create JWT tokens that meet the evolving security trends in application development.
Validating JWT Tokens: Ensuring Security
JWT (JSON Web Tokens) are widely adopted for securing API communications and enabling user authentication in modern applications. To maintain security, effectively validating JWT tokens is pivotal. A well-implemented validation mechanism helps to prevent unauthorized access by ensuring that only legitimate users can interact with protected resources.
First and foremost, validating the signature is a crucial step in JWT security. Each token is generated with a signature that ensures the integrity and authenticity of the claims within the token. Verification is achieved by using a secret key or a public key, depending on whether HMAC (symmetric encryption) or RSA (asymmetric encryption) is utilized. This validation process ensures that the token has not been tampered with since its creation.
Beyond signature verification, validating claims within the JWT is equally important. This includes checking the expiration claim, known as ‘exp’, which denotes when the token will no longer be valid. Failure to adhere to an expired token can lead to significant security vulnerabilities. Another crucial claim to verify is the ‘iss’ (issuer), which confirms that the token originates from a trusted source. Ensuring that these claims meet your application’s expected values fortifies its defenses against impersonation attacks.
As the discussion on security trends expands, it becomes evident that methods such as role-based authentication further enhance this validation process. By implementing access tokens and break down permissions based on user roles, your application can ensure that only authorized individuals access certain functionalities. This approach not only aids in building secure and scalable login systems but also aligns with the trending reasons driving today’s focus on robust authentication practices.
Incorporating APIs such as Google, GitHub, and Facebook for authentication can provide users with a seamless log-in experience, while still maintaining high-security standards through effective token validation. Ultimately, ensuring proper JWT validation is a foundational component of securing your application, safeguarding user data, and maintaining trust in your system.
Implementing Middleware for JWT Authentication
In modern web applications, implementing effective security trends is crucial for ensuring that user data remains protected. One of the prominent methods for secure user authentication is the use of JSON Web Tokens (JWT). Middleware plays an essential role in managing and enforcing JWT authentication throughout the application lifecycle. By placing authentication logic within middleware, developers can easily intercept requests, validate tokens, and enforce user authorization based on roles or privileges.
To implement middleware for JWT authentication, developers begin by creating a middleware function that processes incoming requests. This function checks the presence of a JWT in the request headers, typically in the ‘Authorization’ field. If a token is found, the middleware will decode it using a specified secret key and validate its integrity. This step is critical, as it ensures that only authenticated requests can access sensitive resources and services.
For practical implementation, let’s consider code examples using Node.js with the Express framework. The middleware function can be structured as follows:
const jwt = require('jsonwebtoken');const verifyToken = (req, res, next) => {const token = req.headers['authorization'];if (!token) return res.sendStatus(403); // Forbiddenjwt.verify(token, process.env.JWT_SECRET, (err, decoded) => {if (err) return res.sendStatus(401); // Unauthorizedreq.user = decoded; // Store user info from token for later usenext(); // Proceed to next middleware or route handler});};
This middleware function can be integrated with various routes requiring authentication. The focus on security trends highlights how utilizing JWTs in conjunction with role-based authentication effectively creates a robust authorization system. Additionally, integrating with third-party services such as Google, GitHub, and Facebook APIs can streamline the authentication process further. By employing these security strategies, developers can build secure, scalable login systems essential for today’s applications.
Handling Token Expiration and Refreshing Tokens
In the realm of modern web applications, managing the authentication process efficiently has become crucial, particularly when dealing with token expiration. Security trends such as refresh tokens, access tokens, and role-based authentication have emerged in response to the growing necessity for secure user sessions. The use of JSON Web Tokens (JWT) presents a flexible way to authenticate users while addressing these complexities.
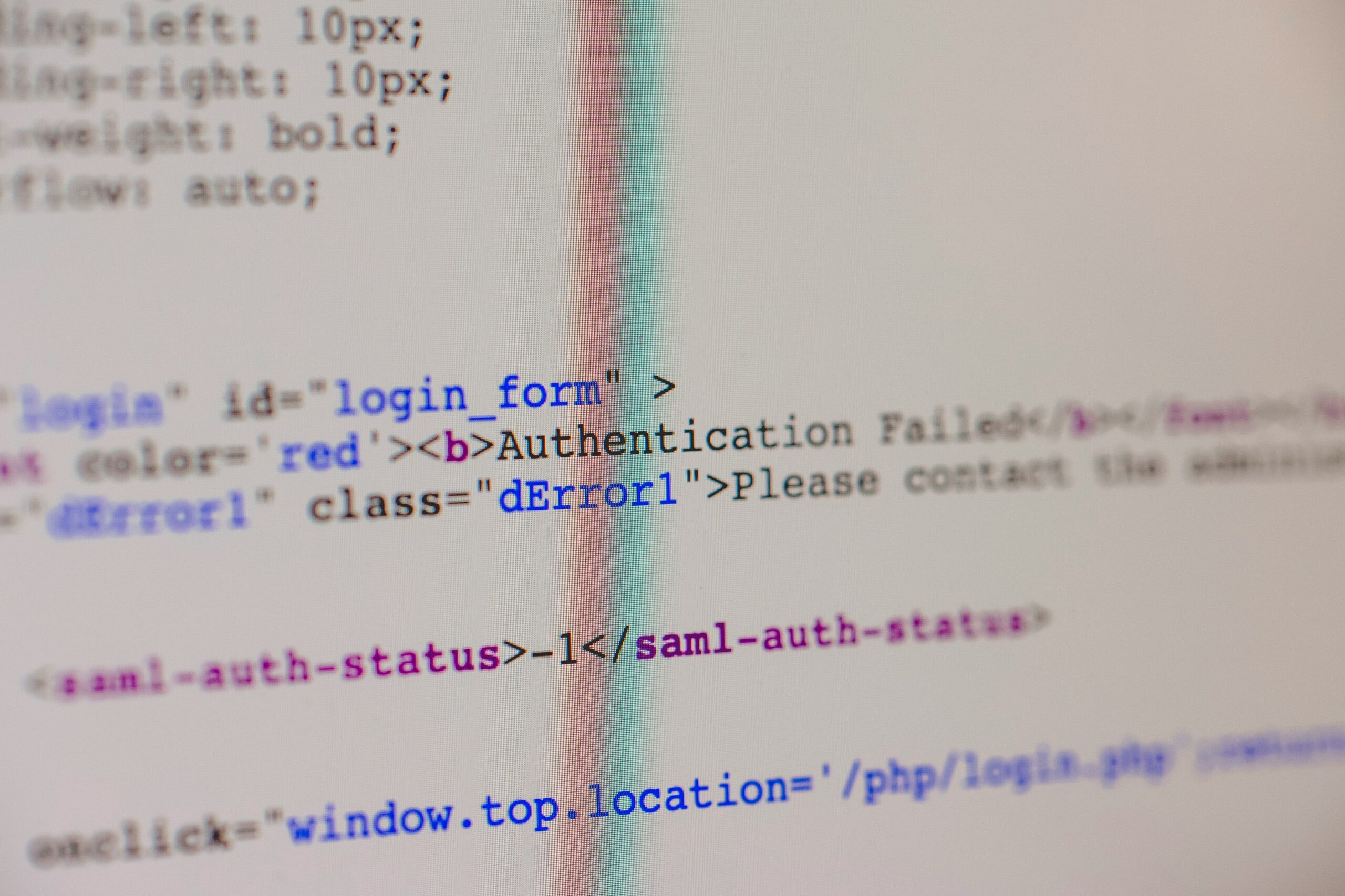
One of the primary challenges with JWT is the limited lifespan of access tokens. This limitation is implemented to reduce the risks associated with token theft. However, it can lead to a poor user experience as users might be frequently required to log back in. Therefore, it is essential to implement a refresh token mechanism. Refresh tokens are long-lived and can be used to obtain new access tokens without initiating the full authentication process again. When building secure, scalable login systems, employing a refresh token strategy ensures that users remain logged in seamlessly while maintaining security.
To implement this strategy effectively, it is advisable to store refresh tokens in secure and HttpOnly cookies. This practice helps mitigate the risk of cross-site scripting (XSS) attacks. Additionally, setting expiration times and revoking refresh tokens during user logout or password changes enhances security. Synchronizing access and refresh tokens is vital, as failing to do so can lead to unauthorized access.
Integration with Google, GitHub, and Facebook APIs allows developers to enrich user authentication experiences while combining role-based authentication strategies. By leveraging these APIs alongside JWT, organizations can apply various roles to users, further expanding their security architecture. Understanding the lifecycle of these tokens and refreshing mechanisms is pivotal in navigating security trends effectively, ensuring that applications remain secure while providing a smooth user experience.
Common Pitfalls and Security Considerations
When implementing authentication with JSON Web Tokens (JWT), it is crucial for developers to be aware of common pitfalls that can lead to security vulnerabilities. These weaknesses often stem from inadequate token storage, poor secret management, and the failure to combat prevalent threats, which can compromise the integrity of a secure and scalable login system.
Firstly, one primary concern is how tokens are stored after they are issued. Using insecure storage solutions, such as local storage in web applications, may expose tokens to cross-site scripting (XSS) attacks. Instead, developers should utilize secure HTTP-only cookies for token storage, as they help mitigate risks associated with client-side attacks. Ensuring that these cookies are marked as secure can prevent sessions from being hijacked over unprotected connections.
Secondly, the management of secrets used to sign JWTs is often overlooked. Developers should generate strong, random secrets and use environment variables to store them rather than hardcoding directly into applications. Regular rotations of these secrets can also enhance security. Additionally, the approach to validating tokens should be robust—developers need to verify the token’s signature and expiry to ensure that the tokens are both authentic and valid.
Also, while building secure systems, it is essential to combat common vulnerabilities such as “JWT None Algorithm” attacks, which can allow an attacker to bypass authentication. Developers must ensure that their systems validate the algorithm specified in the header and reject any requests attempting to utilize the ‘none’ algorithm. Implementing best practices will also include leveraging access tokens effectively and utilizing role-based authentication to ensure appropriate access levels.
In conclusion, understanding the security trends surrounding JWT authentication—focusing on refresh tokens, access tokens, and role-based authentication—is instrumental in avoiding these pitfalls. Awareness of potential vulnerabilities and proactive measures will lead to a more secure implementation and build a trustworthy framework for user authentication.
- sumit singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom