Introduction to Full Stack Development
Full stack development refers to the comprehensive approach of creating web applications that incorporate both frontend and backend technologies. The frontend constitutes the user-facing part of an application, encompassing everything that users interact with directly, such as layouts, designs, and interactivity. In contrast, the backend includes server-side logic, databases, and application programming interfaces (APIs) that manage data and handle requests from the frontend. The integration of these two layers is essential in delivering seamless user experiences, as it ensures that the display and functionality of the application work in concert.
In contemporary web development, the importance of full stack development cannot be overstated. As businesses and users increasingly demand high-performance applications that are responsive and engaging, developers must possess a solid understanding of both the frontend and backend aspects. This proficiency allows for greater flexibility in managing projects, as well as a more holistic view of application architecture. By mastering both layers, developers can more effectively troubleshoot issues, make informed decisions on technology stack choices, and optimize performance across the board.
In recent years, innovative programming languages and technologies have emerged, challenging traditional development paradigms. One such advancement is the use of Rust in combination with WebAssembly (Wasm). Rust is known for its performance and memory safety, making it a compelling choice for backend development, while WebAssembly provides a powerful platform for running code in the browser, enabling high-performance frontend applications. By leveraging full stack development with Rust and WebAssembly, developers can harness the benefits of both technologies to build robust, efficient, and secure web applications that stand out in a competitive market. Embracing this modern stack can significantly enhance the overall development process and user experience.
What is Rust?
Rust is a systems programming language that prioritizes performance, reliability, and productivity. Developed by Mozilla Research, Rust is designed to empower developers to create safe and efficient software without sacrificing speed. A prominent feature of Rust is its emphasis on memory safety, which is achieved through its unique ownership model. This model enforces strict borrowing and lifetime rules, reducing common programming errors such as null pointer dereferences and buffer overflows. By preventing these errors at compile time, Rust ensures a more robust application, making it an appealing choice for both full stack development with Rust and WebAssembly (Wasm).
Another significant aspect of Rust is its support for concurrency. The language’s design allows developers to write multitasking applications without the fear of data races, which are often challenging to manage in other programming languages. Rust’s fearless concurrency model enables developers to leverage multi-core processors effectively, thus improving overall application performance. This capability is especially beneficial for web development, where scalable and responsive applications are essential.
As the demand for high-performance applications increases, Rust has gained traction within the developer community. Its growing ecosystem is characterized by a rich set of libraries and tools that simplify various aspects of programming. Additionally, the active community contributes to improving Rust through open-source initiatives and widespread knowledge-sharing. This has resulted in an ever-expanding library of crates (Rust’s terminology for packages), which facilitates the seamless integration of Rust with other technologies, including WebAssembly. This interoperability expands the scope of full stack development with Rust and WebAssembly (Wasm), allowing builders to create applications that run efficiently in web browsers while maintaining system-level performance. Overall, Rust’s combination of safety, performance, and a supportive community positions it as a compelling option for modern software development.
Understanding WebAssembly (Wasm)
WebAssembly, often abbreviated as Wasm, is a binary instruction format designed to serve as a portable compilation target for programming languages, facilitating high-performance web applications. Developed to offer a secure, fast, and efficient means of running code on the web, Wasm enables developers to execute their applications in web browsers at near-native speed, bridging the gap between high-level programming languages and the browser’s ability to interpret them.
The primary purpose of WebAssembly is to provide a compilation target that allows developers to build web applications using languages like C, C++, and Rust. This offers a significant advantage in terms of performance, particularly for computationally intensive tasks where JavaScript may not suffice. By generating WebAssembly modules, developers can deliver heavy-processing functionalities directly in the browser without relying solely on JavaScript’s performance constraints.
One of the notable benefits of using Wasm is its tendency to improve load times and execution speeds of web applications. Since WebAssembly is a low-level bytecode format, it runs much faster than traditional JavaScript. This is particularly advantageous for applications such as video games, virtual reality, and image editing tools, which require high performance to function optimally. Moreover, Wasm is designed to work across diverse platforms, ensuring that the same compilation works seamlessly on various environments, which enhances portability.
WebAssembly complements full stack development with Rust by leveraging Rust’s memory safety features and performance efficiencies. Rust developers can create robust WebAssembly modules that execute in the browser, benefitting from Rust’s safe concurrency and detection of bugs during compile time. Common use cases for Wasm in web development range from rendering complex graphics using 3D engines to processing large datasets in web applications. Overall, WebAssembly presents a transformative opportunity for developers aiming to harness the full potential of modern web technologies.
Setting Up Your Development Environment
Establishing a suitable development environment is an essential first step for those interested in full stack development with Rust and WebAssembly (Wasm). This setup not only enhances productivity but also facilitates a smoother coding experience. To begin, you will need to install Rust, which is the core programming language you’ll be using. Rust can be easily installed by downloading the Rustup installer from the official Rust website. Once downloaded, run the installer which will set up Rust and its package manager, Cargo, automatically.
After successfully installing Rust, the next step involves incorporating the wasm-pack tool. This utility simplifies the process of building and packaging Rust-generated WebAssembly binaries. You can install wasm-pack using Cargo with the simple command: cargo install wasm-pack
. This command will fetch the tool from the repository and make it available in your environment for building and developing applications that utilize Rust and WebAssembly (Wasm).
Once the necessary tools are installed, configuring your code editor becomes critical. Popular editors, such as Visual Studio Code and IntelliJ Rust, offer excellent support for Rust. Install relevant extensions in your code editor that provide syntax highlighting, code completion, and other valuable features tailored to Rust and Wasm development.
Be prepared to face some common issues during setup. Should you encounter any problems, consult the Rust and wasm-pack documentation, which offers comprehensive troubleshooting guidance. Ensure that your version of Rust is up to date by using the command rustup update
. This will help avoid compatibility issues with various dependencies. Following these steps will facilitate a successful setup, laying a solid foundation for your journey into full stack development with Rust and WebAssembly.
Building a Simple Full Stack Application
Creating a simple full stack application utilizing full stack development with Rust and WebAssembly (Wasm) involves several steps that integrate both backend and frontend technologies effectively. To start, one must establish the backend using Rust, which is known for its performance and safety features. A commonly used framework for this purpose is Actix Web. Begin by creating a new Rust project and adding the necessary dependencies for Actix Web in your Cargo.toml file. This setup will allow you to build a RESTful API capable of handling HTTP requests.
Once the dependencies are in place, you can define your API endpoints. For instance, create a simple endpoint that returns a list of items or users. Using Rust’s powerful type system ensures that your API is not only safe but also efficient, minimizing the risk of runtime errors. Implement appropriate request handlers for various HTTP methods, such as GET and POST, and start your server to test the functionality. At this stage, you can utilize tools like Postman or Curl to validate that your API responds correctly.
After the backend is functional, the next step involves the frontend development with WebAssembly. You will need to convert your Rust code to Wasm, which allows for high-performance execution in the browser. Use the wasm-pack tool to compile the Rust code into a Wasm module. This module can interact with the API you have already created, enabling the frontend to make requests and display the results. In your frontend, you can use JavaScript or a framework such as Yew to create a dynamic interface that consumes your RESTful API, demonstrating a seamless integration of Rust and Wasm.
The combination of Rust’s performance in the backend with the responsiveness of Wasm in the frontend provides a robust architecture for web applications. By following these steps, you can successfully build a simple full stack application that harnesses the power of full stack development with Rust and WebAssembly.
Best Practices in Rust and WebAssembly Development
Full stack development with Rust and WebAssembly (WASM) presents unique opportunities for creating efficient, scalable applications. To effectively harness this power, developers should adhere to best practices that emphasize coding standards, performance optimization, error handling, and maintaining clean architecture.
First and foremost, establishing a robust coding standard is vital. This includes following naming conventions, utilizing consistent formatting, and documenting functions and modules with clear comments. Such practices not only enhance the readability of the code but also facilitate collaboration among team members. When multiple developers are involved, clarity becomes critical to prevent miscommunication and errors that stem from misconceptions regarding code functionality.
Performance optimization is another crucial area in full stack development with Rust and WebAssembly. One effective method is to leverage Rust’s unique memory management capabilities. Developers should strive to minimize memory allocations during runtime, as excessive allocations can lead to performance bottlenecks. Additionally, using Rust’s profiling tools can help identify inefficiencies in the code which can then be optimized accordingly.
Error handling in Rust is robust due to its emphasis on safety. Developers should embrace the use of the Result and Option types, ensuring that potential errors are addressed and appropriately handled. By adopting a proactive approach to error management, one can avoid runtime crashes and improve the overall user experience.
Finally, maintaining clean architecture is paramount. Adopting modular design patterns allows developers to separate concerns and keep components decoupled, leading to easier updates and maintenance. The emphasis on a clear separation of logic can make the codebase more modular and adaptable, allowing for future enhancements without disrupting existing functionality. Overall, these best practices are essential for ensuring that applications built on Rust and WebAssembly remain maintainable and supportable over time.
Testing and Debugging Your Application
Testing and debugging are crucial components of any software development process, and full stack development with Rust and WebAssembly (Wasm) is no exception. When building applications with Rust, developers benefit from a robust testing framework that supports unit testing, integration testing, and documentation tests. This facilitates precise verification of individual components, ensuring that each unit behaves as expected.
To begin, unit tests in Rust are simple to implement by defining test functions annotated with the #[test]
attribute. This integration allows developers to write tests alongside their main code, promoting a cohesive development experience. Additionally, Rust’s built-in testing tools provide various assertions for validating conditions, such as assert_eq!
and assert_ne!
, enhancing the clarity and reliability of the tests.
When applications are compiled to WebAssembly, developers must also consider the environment in which the code executes. Testing within the Wasm environment involves using frameworks such as wasm-bindgen-test
, which allows for running tests in browser contexts as well as headlessly with Node.js. Ensuring that Rust code behaves properly when compiled to Wasm is essential, as discrepancies might arise due to differing environments.
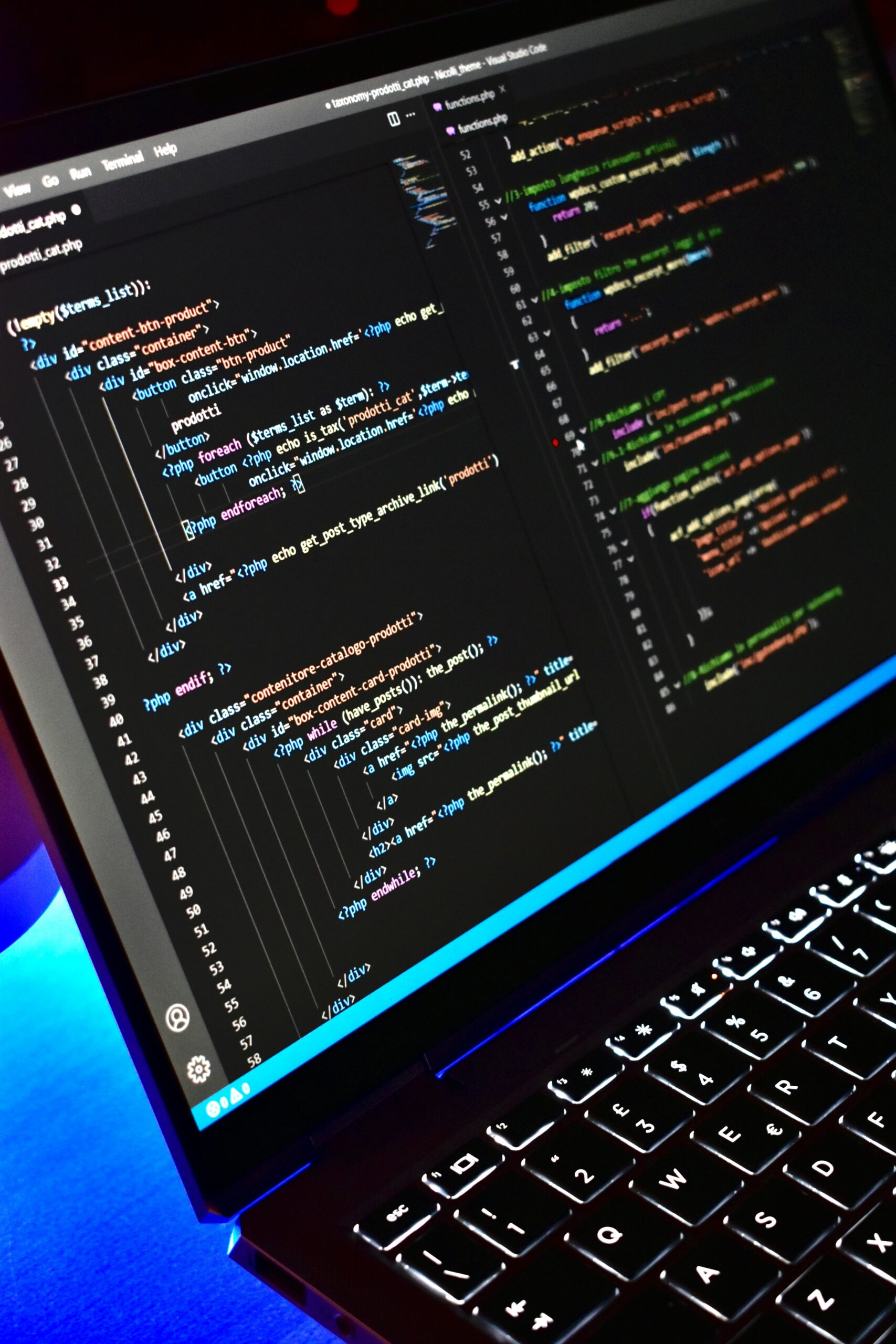
Debugging is equally important in the full stack development process. The combination of Rust and Wasm offers specific tools, such as console_error_panic_hook
, which enhances error reporting in Wasm environments by routing panic messages to the browser console, making it easier to diagnose issues. Additionally, using tools like wasm-toolchain
allows developers to explore and debug their code systematically.
Common problems during the testing and debugging phase with Rust and WebAssembly can include uninitialized variables, incorrect memory handling, and misconfigured dependencies. To address these issues, developers should ensure rigorous error handling within their Rust code and solubilize potential memory pitfalls by utilizing Rust’s ownership model effectively. By implementing solid testing practices and utilizing appropriate debugging tools, developers can significantly enhance the reliability and performance of their full stack applications.
Deploying a Rust and WebAssembly Application
Deploying a full stack application built with Rust and WebAssembly (WASM) requires careful consideration of the web server environment and the configurations necessary to run WebAssembly smoothly. To begin with, selecting an appropriate web server is essential; popular choices include Nginx, Apache, and servers that support WASM directly, such as Actix or Rocket. These servers can effectively handle the static files, including those generated by Rust’s tooling, and can be configured to serve them alongside your application.
In preparation for deployment, it is crucial to ensure that the Rust toolchain is set up correctly to compile your application into WebAssembly. Using tools like wasm-pack
, you can generate optimized output that is suitable for production. This involves ensuring that your code is not only functional but also adheres to best practices in optimization and security, particularly because applications interacting with browsers need to be both efficient and safe from vulnerabilities.
Furthermore, when it comes to deployment platforms, services like Vercel, Netlify, or cloud providers such as AWS and DigitalOcean are ideal as they support Rust applications and have options tailored for hosting WASM files. These platforms simplify the deployment process, providing a continuous integration and deployment pipeline that can automatically build your application and deliver updates seamlessly. Ensure you understand the platform’s specifics regarding routing and handling of static files, as these will determine how your application interacts with users as they navigate through various pages.
Lastly, to ensure a smooth deployment process, it is advised to conduct comprehensive testing and validation of the application in a staging environment before full deployment. This includes load testing to assess performance and checking for compatibility across different web browsers. By following these key steps—selecting an appropriate server, configuring for WebAssembly, choosing a suitable deployment platform, and preparing your application thoroughly—you can successfully deploy a full stack application that leverages the power of Rust and WebAssembly effectively.
Future Trends in Full Stack Development with Rust and WebAssembly
As technology continues to evolve, the landscape of full stack development is set to undergo significant transformations, particularly with the increasing adoption of Rust and WebAssembly (Wasm). The synergy between these two technologies is anticipated to redefine how developers approach building web applications, providing enhanced performance and security features.
One of the most prominent trends is the growing inclination towards integrating Rust in larger and more complex projects. Known for its memory safety and zero-cost abstractions, Rust enables developers to build robust applications that require high performance. As organizations seek to reduce vulnerabilities commonly associated with other languages, the adoption of Rust is expected to rise, especially in sectors demanding high reliability, such as finance and healthcare. This shift will likely encourage more educational resources and community support focusing on full stack development with Rust and WebAssembly, allowing new developers to adopt these technologies with relative ease.
Furthermore, the evolution of WebAssembly will play a crucial role in shaping future development practices. As the specifications of Wasm continue to mature, we can expect enhancements, such as support for multithreading and improved interoperability with JavaScript. These changes will make WebAssembly even more appealing for full stack development as it offers a bridge between high-level scripting languages and low-level assembly-like code, enabling developers to utilize the best features from both realms seamlessly.
Moreover, with the growing interest in performance-critical applications, the trend of building serverless architectures using Rust and WebAssembly is likely to gain momentum. This will facilitate the creation of lightweight web apps that can scale efficiently without incurring significant overhead. Developers should remain vigilant and informed about these emerging trends, as staying updated can position them favorably in a competitive landscape. As we look ahead, full stack development with Rust and WebAssembly promises to catalyze innovation across various industries, paving the way for a new era of versatile and efficient application development.
- Name: Sumit Singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom