Introduction to GraphQL
GraphQL is a modern data query language and runtime that provides a more efficient alternative to traditional REST APIs. Originally developed by Facebook in 2012 and opened to the public in 2015, GraphQL was designed to address the limitations often encountered with RESTful services. It empowers clients by allowing them to request precisely the data they need, thus minimizing over-fetching and under-fetching issues that frequently arise in REST APIs.
In a typical REST API, requests for data can lead to the transfer of large amounts of unnecessary information, as endpoints are structured around fixed data shapes. This can result in clients receiving more data than required for a specific operation. Conversely, GraphQL operates on a flexible model where clients specify their desired data structure through a single endpoint. This dynamic querying enables clients to retrieve nested resources in a single request, significantly enhancing performance and reducing the number of network calls required.
The core functionality of GraphQL revolves around its type system, which defines the capabilities of an API. Developers can create queries that precisely express their data needs, a valuable feature when building applications that require better performance for mobile environments where bandwidth and data usage may be limited. Moreover, GraphQL’s schema provides clear documentation of available data types and relationships, making it easier for developers to understand how to interact with the API effectively.
In essence, GraphQL represents a paradigm shift in how clients interact with APIs, favoring efficiency and developer experience. By enabling flexible queries, empowering clients to request only the necessary data, and thus optimizing overall performance, GraphQL positions itself as a strong alternative to traditional REST APIs, particularly in the context of modern applications developed using the MERN stack.
Understanding MERN Stack
The MERN stack is a powerful set of technologies that together form a robust framework for building modern web applications. The acronym MERN stands for MongoDB, Express.js, React, and Node.js, each of which plays a crucial role in creating dynamic and efficient applications. Understanding these components is vital for developers seeking to replace traditional REST APIs with GraphQL in their projects.
MongoDB serves as the database layer of the MERN stack. It is a NoSQL database that stores data in JSON-like documents, making it easy to create, read, update, and delete data. Its flexibility allows developers to work with varying data structures without the need for a predefined schema, which enhances agility in application development. In a typical web application, MongoDB efficiently handles data storage and retrieval, contributing to better performance for mobile and web interfaces.
Express.js operates as the back-end framework that runs atop Node.js. It simplifies the creation of server-side applications by providing a minimalist structure and robust routing capability. By using Express.js, developers can set up APIs without the overhead of complex configurations. It facilitates the implementation of middleware functions that can modify requests or responses, thus streamlining the data handling process necessary for effective communication between the client and server.
React forms the front-end component of the MERN stack, focusing on building user interfaces. Its component-based architecture enables developers to create reusable UI elements that improve code maintainability and reduce development time. React’s virtual DOM enhances application performance, ensuring that updates to the user interface are both fast and efficient. This efficiency is critical in providing an optimal experience for mobile users.
Finally, Node.js is the runtime environment that allows JavaScript to be executed on the server side. It is built on Chrome’s V8 JavaScript engine and provides a non-blocking, event-driven architecture that enhances scalability. Node.js allows developers to utilize JavaScript throughout the entire stack, promoting skills reuse and a more cohesive development experience. Through these four technologies working in unison, the MERN stack offers a high-performance framework ideal for web applications, making it an excellent candidate for integration with GraphQL to enhance data management capabilities.
Challenges of Traditional REST APIs
Traditional REST APIs have been a popular choice for backend development for many years, but they come with inherent challenges, particularly in the context of data fetching. One of the most significant issues is the phenomenon of over-fetching and under-fetching data. Over-fetching occurs when an application retrieves more data than it actually needs for a particular operation. For instance, if a developer requires only a user’s name and email address but the API endpoint delivers a full user profile with additional fields such as address, phone number, and profile picture, this can lead to unnecessary bandwidth usage and a decrease in application performance, particularly on mobile devices where efficient data use is critical.
Conversely, under-fetching happens when an API does not provide all the required data in a single request, forcing the client to make multiple calls to gather the necessary information. This can lead to increased latency and a poor user experience, especially for mobile users who may be dependent on limited bandwidth and high latency environments. Consider an app loading a list of products, where each product requires additional details fetched via separate API calls. This not only burdens the system with excessive requests but also results in longer loading times, diminishing the overall performance for mobile.
These challenges illustrate why many developers are seeking alternatives to traditional REST APIs. The architectural limitations can hinder scalability and complicate the development process, particularly as applications become more complex. As the demand for responsive and efficient applications continues to grow, exploring solutions such as GraphQL can provide a remedy to these common pitfalls. By addressing over-fetching and under-fetching issues, developers can enhance performance and improve the overall user experience in their applications.
Benefits of Using GraphQL
GraphQL has emerged as a compelling alternative to traditional REST APIs, particularly within the MERN stack. One of the foundational benefits of using GraphQL is its flexibility in formulating queries. In contrast to REST, where predefined endpoints limit how data can be extracted, GraphQL allows clients to request exactly the data needed. This means that applications can avoid over-fetching or under-fetching data, resulting in better performance for mobile devices. Such precision is essential in fostering efficient data retrieval, ultimately leading to enhanced user experiences.
Another significant advantage of GraphQL is the reduction in the number of API calls. In REST API architecture, developers often have to make multiple requests to different endpoints to gather related information. This can be cumbersome and lead to latency issues, particularly in mobile contexts where bandwidth may be limited. By contrast, GraphQL enables developers to compile multiple resource requests into a single query, thus decreasing the overall number of API calls. This is particularly beneficial for mobile applications that rely on quick, efficient data loading to maintain responsiveness.
Furthermore, GraphQL provides the ability to aggregate multiple related data sources in a single request. This feature allows developers to draw from various APIs or databases simultaneously, streamlining the data-fetching process. In practical scenarios, such as e-commerce applications, this means a single query can fetch product details, reviews, and inventory status, all in one go. The efficient data fetching pattern of GraphQL not only leads to better overall performance for mobile applications but also simplifies the client-side code and enhances maintainability.
Implementing GraphQL in the MERN Stack
Integrating GraphQL into a MERN stack application can significantly enhance data fetching capabilities, resulting in better performance for mobile environments. To start, ensure that you have Node.js installed on your machine, as it will serve as the runtime for your server-side application. You’ll also need MongoDB for the database layer. Begin by creating a new directory for your project and running npm init -y
to initialize a new Node.js project.
Next, install the necessary libraries to facilitate the GraphQL server setup. You will require express
, apollo-server-express
, and graphql
. Use the following command:
npm install express apollo-server-express graphql mongoose
Once the installation is complete, create a new file named server.js
. In this file, you will set up the Express server and implement Apollo Server. First, import the required modules:
const express = require('express');const { ApolloServer } = require('apollo-server-express');const mongoose = require('mongoose');
Next, connect to your MongoDB database using Mongoose. Inside server.js
, initiate the connection:
mongoose.connect('your_mongodb_connection_string', { useNewUrlParser: true, useUnifiedTopology: true });
Now, define your GraphQL schema. A simple schema for a user model can look something like this:
const typeDefs = gql`type User {id: ID!username: String!email: String!}type Query {users: [User]}`;
After defining the schema, implement the resolvers, which essentially define how to fetch the data for your queries:
const resolvers = {Query: {users: async () => await User.find(),},};
With the schema and resolvers defined, create the Apollo Server instance and apply it to your Express app:
const server = new ApolloServer({ typeDefs, resolvers });server.applyMiddleware({ app });
Finally, start the server by adding the following line, specifying the desired PORT:
app.listen({ port: 4000 }, () =>console.log(`🚀 Server ready at http://localhost:4000${server.graphqlPath}`));
In this setup, you have successfully integrated GraphQL into your MERN stack application. The data fetching capabilities provided by GraphQL will ensure a better performance for mobile users, allowing them to interact seamlessly with your application. This foundational setup can serve as a springboard for further enhancements and optimizations as your application evolves.
Querying Data with GraphQL
GraphQL introduces a flexible and efficient approach to querying data that minimizes data retrieval while maximizing relevance. Unlike traditional REST APIs that often require multiple endpoints to fetch related data, GraphQL enables developers to obtain all required information through a single query, streamlining the process and improving performance for mobile applications.
To create queries in GraphQL, a basic understanding of its syntax is essential. A simple query can be constructed to retrieve specific fields from a data type. For instance, if we want to fetch information about a user, such as their name and email, the query would look as follows:
{user(id: "1") {nameemail}}
This query clearly specifies the required fields: ‘name’ and ’email,’ ensuring that only the necessary data is fetched, and nothing extraneous is retrieved. This tailored request significantly enhances the data transfer process, particularly important for applications with users on mobile devices, where bandwidth and speed are often concerns.
Moreover, GraphQL supports complex queries with nested fields, allowing users to retrieve related data in a single request. For example, if we need not only the user’s name and email but also their posts, we can extend our query:
{user(id: "1") {nameemailposts {titlecontent}}}
This nested structure showcases how GraphQL consolidates data into one coherent structure, minimizing the need for multiple requests. Additionally, variables can be utilized to make our queries more dynamic, such as defining a user ID as a variable:
query GetUser($id: ID!) {user(id: $id) {nameemail}}
By incorporating variables, developers can create reusable and adaptable queries that enhance overall efficiency and flexibility in data retrieval, contributing to better performance for mobile applications.
Mutating Data with GraphQL
In the realm of GraphQL, data mutations represent a crucial aspect of handling dynamic operations, particularly in the context of the MERN stack. Unlike traditional REST APIs where each action often requires a separate endpoint, GraphQL allows developers to perform create, update, and delete operations through structured mutation queries. This flexibility offers a more efficient approach for better performance for mobile applications, ensuring that data manipulation is streamlined.
To create a record, a specific mutation query must be crafted. Typically, this involves defining the mutation in the schema. For instance, to create a new user, you might define a mutation like this:
mutation {createUser(input: {name: "John Doe", email: "john@example.com"}) {idnameemail}}
In this example, the createUser
mutation allows users to submit new data as an input object, and in return, it provides designated fields such as id
, name
, and email
, thereby ensuring a clear structure in the response.
Updating records similarly involves constructing a mutation that specifies the fields to modify. For example, to update a user’s email, the following mutation query is used:
mutation {updateUser(id: "1", input: {email: "john.new@example.com"}) {idnameemail}}
This illustrates how a single mutation can effectively manage the update process while maintaining a straightforward syntax. Lastly, deleting records also follows a similar pattern. For instance:
mutation {deleteUser(id: "1") {idname}}
Here, the deleteUser
mutation allows for the removal of the specified user, reinforcing the comprehensive capabilities of GraphQL for data manipulation. Overall, these mutation principles not only empower developers but also contribute to better performance for mobile, allowing apps to function with a greater degree of efficiency and responsiveness.
Real-World Applications of GraphQL in MERN Stack
The transition from traditional REST APIs to GraphQL within the MERN stack has increasingly gained traction across various industries, demonstrating significant advantages in performance and efficiency. Several notable applications have successfully utilized GraphQL to enhance user experience while optimizing data fetching processes.
One prominent example is in the e-commerce sector, where companies have adopted GraphQL to streamline their product catalog searches. By allowing queries that return only the required fields, businesses can significantly reduce the amount of data transmitted, leading to faster load times on mobile devices. This better performance for mobile not only improves user satisfaction but also boosts conversion rates, providing a competitive edge in a saturated marketplace.
Another exemplary use case can be found in the healthcare industry. Various health tech startups have implemented GraphQL to manage patient records and streamline inter-user communications. The ability to request and receive specific patient information without overwhelming the user interface has been pivotal; healthcare professionals can access detailed information quickly on mobile devices, enhancing patient care by facilitating timely and informed decisions.
Moreover, in the realm of social media applications, platforms integrating GraphQL within their MERN stack have reported improved interaction metrics. By leveraging GraphQL’s flexibility to request various related data sets in a single call, applications can present richer and more relevant content to users, thus enhancing overall engagement. This clear focus on user experience aligns well with the trend towards mobile-first approaches in application development.
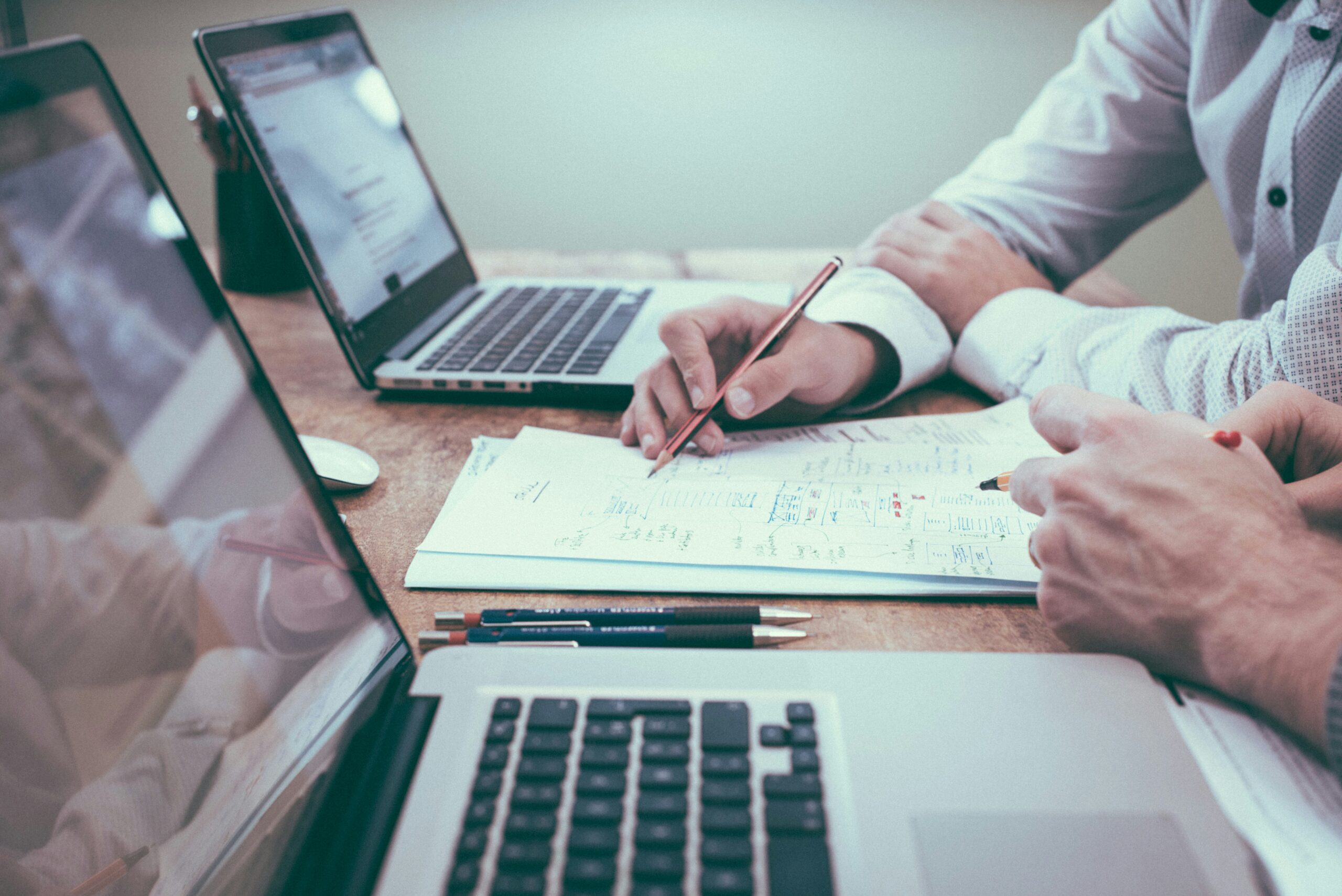
In summary, across various sectors such as e-commerce, healthcare, and social media, the implementation of GraphQL within the MERN stack has proven beneficial. By ensuring better performance for mobile applications and dramatically improving user experiences, businesses are now positioned to thrive in an increasingly digital world.
Conclusion and Future Trends
The transition from traditional REST APIs to GraphQL within the MERN stack embodies a significant evolution in web development. One of the primary advantages of GraphQL is its efficiency in data retrieval. Unlike REST, where multiple endpoints may be required to fetch related data, GraphQL’s single query approach allows developers to retrieve precisely what they need, optimizing performance and enhancing the experience for mobile users. This tailored data-fetching capability reduces the amount of redundant data transferred over networks, ultimately leading to a more responsive application.
Moreover, the flexibility offered by GraphQL is noteworthy. Developers can define the structure of their queries, ensuring that the data returned aligns perfectly with the application’s requirements. This adaptability is particularly beneficial in the modern landscape, where mobile devices vary significantly in capabilities and screen sizes. By providing an API that caters to these diverse needs, GraphQL improves overall user experience and ensures better performance for mobile applications.
Looking ahead, the future of API development appears to be heavily influenced by GraphQL’s growing popularity. As businesses increasingly utilize microservices architectures, the need for a more dynamic and efficient method of data retrieval from various sources will become paramount. Integrating GraphQL within these environments will likely streamline processes, allowing for swift adaptations to ever-changing business needs.
Furthermore, the community around GraphQL is robust and continuously expanding. This ensures ongoing support and innovation, making it a priority for developers seeking to enhance their applications. Coupled with the advancements in technology, such as improved caching mechanisms and increased adoption of serverless architectures, GraphQL is well-positioned to reshape the landscape of web development in the years to come.
- Name: Sumit Singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom