Introduction to Programming Paradigms
Programming paradigms are fundamental styles or approaches to programming that dictate how developers conceptualize and structure software solutions. They encompass various methodologies, each offering distinct benefits and challenges. Understanding programming paradigms is crucial for software developers, as it influences how they design, implement, and maintain applications. By recognizing shifts in these paradigms, one can grasp the broader context of software development and its response to evolving technological demands.
The evolution of programming paradigms demonstrates a progressive shift from procedural programming, characterized by sequences of statements or instructions, to more complex models such as object-oriented, functional, and ultimately AI-driven development. Procedural programming focuses on writing instructions in a linear fashion and primarily revolves around functions and procedures. While this paradigm laid the groundwork for structured programming, it has limitations in representing complex systems and facilitating maintainability in large-scale projects.
As software applications grew in complexity, the need for more sophisticated approaches became apparent. The emergence of object-oriented programming introduced concepts like encapsulation, inheritance, and polymorphism, which allowed for better organization and reuse of code. Functional programming, on the other hand, emphasized immutability and first-class functions, enabling developers to model computations more intuitively.
Today, the landscape has shifted again with the rise of AI-driven development, which leverages machine learning and artificial intelligence techniques to enhance coding practices. This contemporary paradigm focuses on improving automation, predictive capabilities, and human-computer interaction. The transition from procedural to AI-driven methodologies not only illustrates the dynamism of programming paradigms but also emphasizes the importance of adapting to changing technological landscapes. Comprehending this evolution is vital, as it drives innovation and efficiency in software development.
Overview of Procedural Programming
Procedural programming is a fundamental programming paradigm that emerged in the mid-20th century, significantly influencing the development of software engineering. At its core, procedural programming revolves around the concept of procedure calls, dividing a program into a collection of functions or procedures that perform specific tasks. This modular approach allows for organized and systematic coding, enhancing readability and maintainability of code.
The foundation of procedural programming is built upon key concepts such as functions, control structures, and data management. Functions are the building blocks that encapsulate reusable code, promoting the principle of “write once, use many times.” This modularity not only streamlines code development but also facilitates debugging and testing. Control structures, including loops and conditional statements, enable the flow of execution to be dictated by specific conditions, leading to more dynamic and responsive programs.
Historically, procedural programming languages such as C and Pascal played a pivotal role in shaping the landscape of software development. Introduced in the early 1970s, C offered low-level access to memory, making it a robust choice for systems programming and influenced numerous later languages. Pascal, developed in the late 1960s, emphasized teaching programming principles and structured programming, establishing a strong educational foundation that has impacted numerous programmers worldwide. The advent of these languages marked a shift in how developers approached programming tasks, leading to more systematic methodologies.
Over the years, procedural programming has proven to be highly effective, serving as a stepping stone to more advanced paradigms. While newer methodologies such as object-oriented and functional programming have emerged, the influence of procedural programming remains significant, having laid the groundwork for subsequent innovations in programming languages and practices. As the landscape continues to evolve, the foundational elements of procedural programming still provide essential concepts that enhance our understanding of programming paradigms.
The Rise of Object-Oriented Programming
Object-oriented programming (OOP) emerged in the 1960s and 1970s as a significant advancement in the evolution of programming paradigms, building on the limitations inherent in procedural programming. One of the key principles of OOP is encapsulation, which involves bundling data and the methods that operate on that data into single units known as objects. This encapsulation allows for more robust data protection and promotes modular code development, enabling developers to create systems that are more manageable and easier to understand.
Another fundamental principle of OOP is inheritance. This concept allows developers to create new classes that inherit attributes and behaviors from existing classes. By reusing code, inheritance reduces redundancy and encourages a hierarchical organization of classes, which fosters an efficient means of extending functionality without modifying existing code. For instance, a base class `Vehicle` might have derived classes `Car` and `Truck` that inherit common features while introducing specific properties unique to each type of vehicle.
Polymorphism, the third key principle, enables objects to be treated as instances of their parent class while allowing for method overriding in derived classes. This flexibility allows programmers to write more generic and reusable code. For example, one can create a method that accepts a `Vehicle` object and, regardless of whether it is a `Car` or `Truck`, invokes the appropriate behaviors. Such capabilities enhance the overall design of software and contribute to more scalable applications.
Popular programming languages that embody these OOP principles include Java and C++, both widely used in the industry for developing applications that require robust architecture and scalability. Through the adoption of object-oriented programming, developers have significantly improved code organization and maintainability, marking a notable transformation in the landscape of programming paradigms.
Functional Programming and its Influence
Functional programming is a programming paradigm that emphasizes the use of functions to create software. This approach significantly contrasts with more traditional paradigms, such as procedural and object-oriented programming (OOP), by promoting a different perspective on computation and data manipulation. At its core, functional programming relies on fundamental concepts such as first-class functions, immutability, and higher-order functions, which collectively facilitate a style of programming that is declarative rather than imperative.
First-class functions are a crucial element of functional programming, allowing functions to be treated as first-class citizens. This means that functions can be assigned to variables, passed as arguments to other functions, and returned as values, promoting a more abstract way of coding. Immutability is another cornerstone of this paradigm; once a data structure is created, it cannot be altered. This idea tackles the complexities associated with state management in procedural and OOP contexts, effectively minimizing side effects and enhancing the reliability of code.
Higher-order functions, which can accept functions as parameters or return them as results, further exemplify the flexibility and power of functional programming. These functions enable developers to compose operations in ways that make code more expressive and concise. Historically, languages like Lisp have laid the groundwork for functional programming. Lisp’s reliance on recursive functions and list processing paved the way for newer languages. Haskell took this paradigm further by introducing strong static typing and lazy evaluation, while Scala merges object-oriented and functional programming principles, supporting both paradigms. The evolution from procedural programming to functional programming marks a pivotal shift in software development, reflecting the need for better abstractions and scalability.
The Impact of Scripting and Declarative Languages
The emergence of scripting and declarative programming languages marked a significant evolution in the landscape of programming paradigms. Unlike traditional procedural languages that emphasize a step-by-step approach to problem-solving, scripting languages like Python and Ruby provide a more concise and expressive syntax. This shift allowed developers to achieve complex tasks with fewer lines of code, enhancing efficiency and readability. The simplicity afforded by these languages made them highly accessible to a broader audience, allowing non-programmers to engage in software development and automation.
Python, in particular, has gained immense popularity due to its versatility and ease of learning. Its application spans from web development to data analysis and artificial intelligence, showcasing the adaptability that scripting languages offer. Similarly, Ruby, known for its elegant syntax, revolutionized web development with frameworks like Ruby on Rails, which streamlined the process of building robust applications while following convention over configuration principles. These languages have facilitated a transition from procedural to more agile and dynamic methods of writing code.
In addition to scripting languages, declarative programming has risen to prominence, particularly with the advent of SQL. Declarative languages focus on what the outcome should be rather than how to achieve it. This approach allows developers to specify desired results, and the underlying system determines the best way to produce those outcomes. Such paradigms have become instrumental in managing databases and querying information efficiently. Moreover, the rapid growth of web development necessitated new programming practices that favored these languages, ultimately reshaping how applications are built and maintained in today’s technology-driven world.
The Era of Concurrent and Parallel Programming
As software demands grow and the prevalence of multi-core processors rises, the significance of concurrent and parallel programming paradigms has become increasingly pronounced. These paradigms facilitate the capacity to execute multiple processes simultaneously, thereby optimizing performance and resource utilization. The transition from singular, procedural programming approaches to these advanced methodologies reflects an essential evolution within the programming landscape.
At the core of concurrent programming are threads, which represent independent paths of execution within a single process. By allowing different sections of code to run concurrently, threads maximize CPU usage and enhance responsiveness, particularly in user-facing applications. Asynchronous programming further complements this by permitting operations to occur without blocking the main execution thread. This allows for more efficient handling of tasks such as I/O operations where waiting for responses could detrimentally impact user experience.
Frameworks and libraries have emerged to simplify the complexities associated with concurrent and parallel execution. Notably, technologies like Java’s Fork/Join framework and Python’s asyncio offer developers tools to implement parallelism effectively without delving into low-level threading intricacies. These advancements not only reduce development time and effort but also contribute to writing cleaner, more maintainable code.
Real-world applications underscore the practical advantages of these programming paradigms. In data processing, for instance, breaking down tasks into smaller, parallelizable chunks allows for significant improvements in performance, particularly when handling large datasets. Additionally, applications in machine learning and big data benefit immensely from parallel execution, enhancing computational speed and enabling the handling of more extensive datasets and complex algorithms.
The evolution towards concurrent and parallel programming has fundamentally transformed how developers approach problem-solving, meeting the challenges of an increasingly concurrent computing environment with greater adaptability and efficiency. As technology continues to advance, the relevance of these paradigms will undoubtedly expand, reinforcing their place in the programming sphere.
The Emergence of AI-Driven Development
The programming landscape has witnessed significant transformations over the decades, and the emergence of AI-driven development stands as the latest milestone in this evolution. This modern paradigm highlights the integration of advanced technologies such as machine learning, neural networks, and automation tools to redefine how software is developed. AI-driven development empowers programmers to implement intelligent algorithms capable of analyzing vast amounts of data, learning from patterns, and making predictive decisions. This shift not only augments the developers’ capabilities but also enhances overall efficiency.
One of the most critical aspects of AI-driven development is its ability to automate repetitive coding tasks. Tools and platforms leveraging AI assist developers by providing code suggestions, automatically generating code snippets, or identifying bugs in real time. Popular integrated development environments (IDEs) are increasingly incorporating features powered by AI to facilitate these improvements. For instance, GitHub’s Copilot utilizes natural language processing, allowing developers to compose entire functions simply by inputting a description of what they require. This form of system is revolutionizing coding practices, as it minimizes manual effort and reduces the likelihood of human error.
Additionally, machine learning algorithms can assess previous projects, iteratively improving their suggestions based on user feedback and performance outcomes. This continuous learning model opens doors for greater customizations, catering to specific programming needs and preferences. Furthermore, frameworks such as TensorFlow and PyTorch harness neural networks to provide robust solutions for complex coding challenges, making it easier to create sophisticated applications.
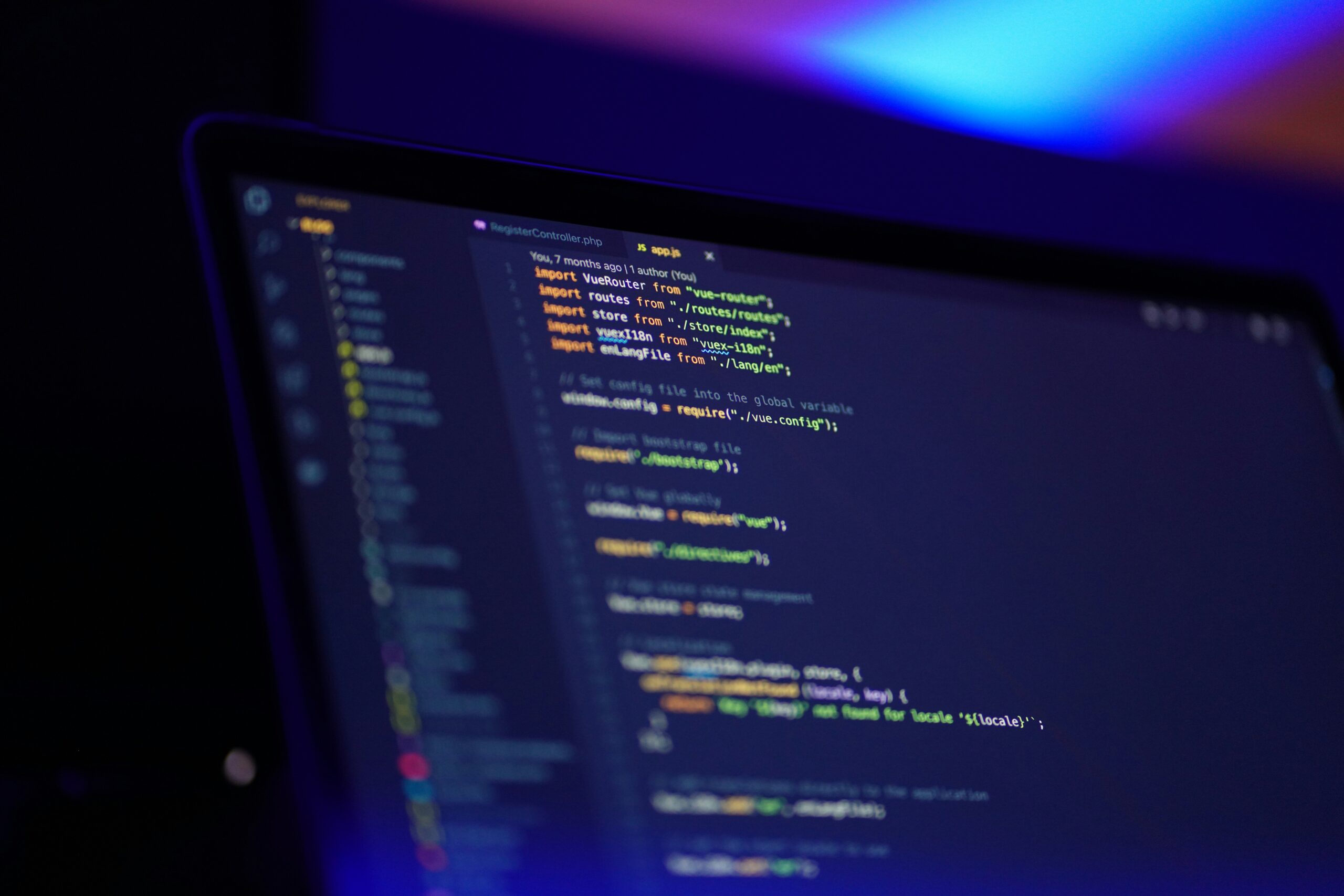
As we stand at the intersection of innovation and practicality, AI-driven development is not merely an incremental change but a transformational force driving the evolution of programming paradigms. This paradigm shift brings forth the promise of lower development costs, faster turnaround times, and high-quality software, further solidifying its place in the future of programming.
Comparing Programming Paradigms: A Shift in Mindset
The evolution of programming paradigms reflects a significant shift in how developers approach problem-solving and software design. Each paradigm, from procedural to object-oriented and into the realm of AI-driven development, embodies unique philosophies that influence both coding practices and the overall development workflow. Procedural programming, one of the earliest paradigms, is centered around a sequence of statements or instructions to be executed. This approach fosters a linear mindset, making it easier for developers to plan and structure tasks in a clear and systematic manner.
In contrast, object-oriented programming (OOP) introduces a model that focuses on objects and the interactions between them. This paradigm encourages developers to think in terms of real-world entities, promoting encapsulation, inheritance, and polymorphism. While OOP can lead to more organized and manageable code, it also brings complexity, as understanding the relationships and hierarchies within the objects requires a deeper conceptual shift.
As software development progresses toward more advanced techniques, such as functional programming and AI-driven paradigms, the cognitive demands on developers continue to evolve. Functional programming emphasizes pure functions and immutability, enabling developers to reason more effectively about code and leading to fewer side effects. However, this can also introduce a steep learning curve for those accustomed to imperative styles.
AI-driven development represents the most recent shift, wherein programmers leverage artificial intelligence to address challenges in innovative ways. This paradigm fosters creativity, as it enables the exploration of previously unimaginable solutions, ultimately extending the boundaries of traditional software development. However, adopting AI-driven methodologies brings its own set of challenges, particularly in terms of ethical considerations and the need for interdisciplinary knowledge.
Therefore, when deciding on the most suitable programming paradigm for a given project, it is crucial for developers to assess not only the advantages and limitations inherent in each approach but also their own preferences and workflows. This critical evaluation allows for optimized problem-solving techniques and more efficient software design.
The Future of Programming Paradigms
The landscape of programming paradigms continues to evolve, driven by technological advancements and changing developer needs. Emerging trends such as low-code and no-code development platforms are gaining momentum, enabling a broader spectrum of users, including those without extensive programming expertise, to contribute to software creation. This shift toward accessible development tools suggests a future where programming is more democratic, allowing non-developers to partake in the development process. Such trends may redefine what it means to be a programmer, shifting the focus from pure coding skills to understanding logic, user experience, and problem-solving abilities.
Advancements in artificial intelligence (AI) play a crucial role in shaping the future of programming paradigms. AI-driven development tools are beginning to assist developers by automating repetitive tasks, generating code snippets, and even suggesting entire functions based on high-level descriptions. As machine learning techniques continue to improve, we may witness an increase in AI’s capability to understand and refine code, ultimately boosting developer productivity and enabling more complex projects to be tackled with ease. This transition indicates a potential hybridization of programming paradigms where traditional methods interweave with intuitive AI tools, creating a collaborative computing environment.
Furthermore, expert opinions indicate that the rise of hybrid paradigms, where procedural code and AI tools coexist, may become more prevalent. As the software development field progresses, it’s crucial for developers to adapt and embrace these changes. Continuous learning and reskilling will be vital in order for professionals to thrive within this evolving landscape. By integrating low-code solutions and harnessing AI, developers can focus on more strategic tasks, enhancing software functionality, user experience, and responsiveness. Overall, the paths laid out by these emerging trends offer exciting prospects for the future of programming paradigms.
Read more blogs https://eepl.me/blogs/
For More Information and Updates, Connect With Us
- Name: Sumit Singh
- Phone Number: +91-9835131568
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom